API Design: Best Practices for Building Scalable Interfaces
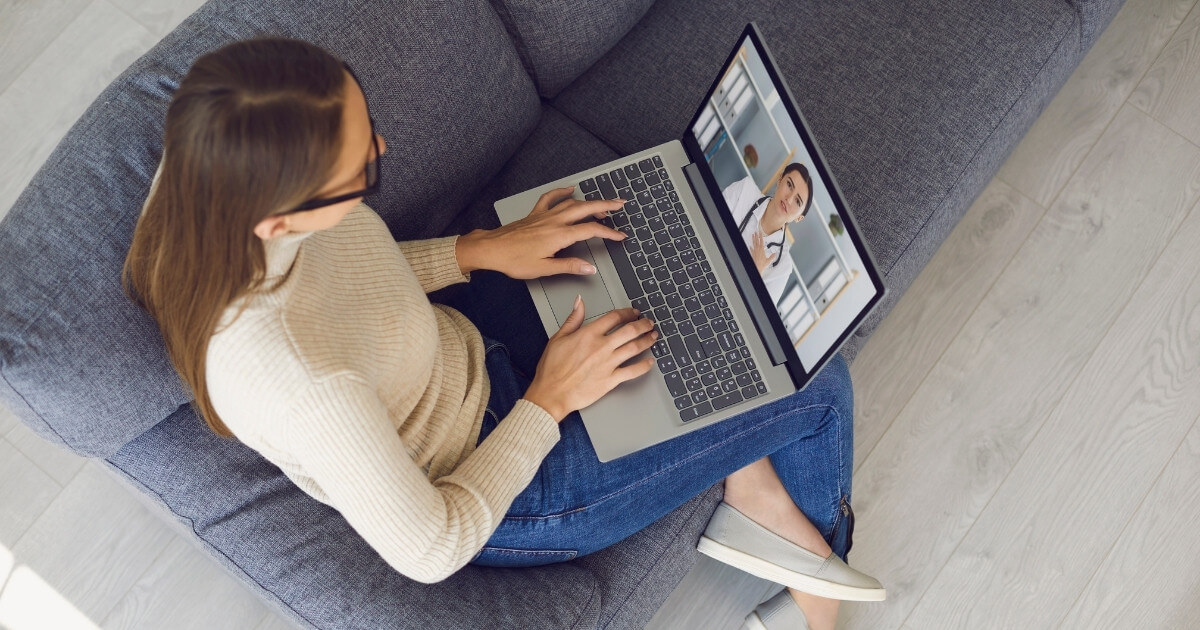
Good API design follows standard patterns and practices that have evolved over time. REST principles guide many modern APIs, providing a structured way to handle data and operations. These principles help create APIs that are easy to use, maintain, and scale.
API design choices impact both developers and end users. Clear naming, proper error handling, and thoughtful documentation make APIs more user-friendly. Security and performance considerations must be built into the design from the start.
Key Takeaways
- Clear API design patterns create a solid foundation for application development
- REST principles guide effective API structure and resource handling
- Proper security and performance measures strengthen API reliability
Understanding API Design
API design creates rules and standards for how software applications communicate with each other. Good API design leads to better integration, fewer errors, and happier developers.
API Development Lifecycle
APIs start with planning and requirements gathering. Teams define the API's purpose, target users, and key functionality.
Design comes next, with teams creating detailed specifications for endpoints, data models, and authentication.
Development involves writing code, building the API endpoints, and implementing security measures.
Testing checks for bugs, performance issues, and security holes through unit tests and integration testing.
Deployment moves the API to production servers with proper monitoring and scaling in place.
Maintenance includes updates, bug fixes, and version control to keep the API running smoothly.
API Architectures
Monolithic Architecture
- Single codebase handles all API functions
- Simple to develop and deploy
- Can become complex as it grows
Microservices Architecture
- Split into small, independent services
- Each service handles specific functions
- Easier to scale and maintain
Layered Architecture
- Separates concerns into distinct layers
- Presentation, business logic, and data layers
- Helps organize complex APIs
API Paradigms: REST and GraphQL
REST APIs use standard HTTP methods like GET, POST, PUT, and DELETE. They work with resources and endpoints that map to specific URLs.
GraphQL offers more flexibility with a single endpoint. Clients can request exactly the data they need, reducing over-fetching and under-fetching.
REST excels in simple CRUD operations and caching. GraphQL shines when clients need complex data from multiple sources.
Protocols and Data Formats
Common Protocols:
- HTTP/HTTPS for web APIs
- WebSocket for real-time data
- MQTT for IoT devices
Data Formats:
- JSON: Most popular, easy to read
- XML: More structured, used in enterprise
- Protocol Buffers: Efficient binary format
APIs need clear documentation about their protocols and formats. This helps developers integrate correctly and reduces support issues.
API Design Principles
Good API design follows essential principles that create reliable, secure, and easy-to-use interfaces. These principles guide developers in building APIs that serve users effectively while maintaining strong technical standards.
Simplicity and Flexibility
APIs need clear, predictable patterns that make them easy to learn and use. Each endpoint should have a single, well-defined purpose.
Keep method names short and descriptive. Use consistent naming across all endpoints to help developers understand the API faster.
Make the API flexible enough to grow with future needs. Design endpoints to handle different data formats and allow for optional parameters when needed.
Break complex operations into smaller, focused endpoints. This makes the API easier to maintain and reduces the chance of errors.
Security and Performance
Use rate limiting to prevent API abuse. Set appropriate limits based on user roles and expected usage patterns.
Implement proper authentication methods like API keys or OAuth tokens. Never expose sensitive data in URLs or error messages.
Cache responses when possible to improve speed. Set proper cache headers to help clients manage their data effectively.
Monitor API performance and set clear response time goals. Optimize database queries and minimize payload sizes to meet these goals.
Principles of RESTful APIs
Design APIs around resources rather than actions. Use nouns for endpoint names and HTTP methods to indicate actions.
Follow standard HTTP status codes. Return 200 for success, 201 for creation, 400 for bad requests, and 404 for missing resources.
Make APIs stateless. Each request must contain all information needed to complete the operation.
Use proper HTTP methods:
- GET: Read resources
- POST: Create new resources
- PUT: Update existing resources
- DELETE: Remove resources
Version your APIs to maintain compatibility as they evolve. Include version numbers in the URL or headers.
API Specification Tools
API specification tools help teams create clear documentation and contracts for their APIs. These tools make it easier to design, test, and share API details across development teams.
OpenAPI and Swagger
OpenAPI Specification, previously known as Swagger, is the most widely used standard for describing REST APIs. It creates machine-readable documentation that defines API endpoints, request methods, and data formats.
Swagger offers a complete set of development tools built around the OpenAPI standard. These tools include Swagger Editor for writing specifications and Swagger UI for creating interactive API documentation.
Teams can write OpenAPI specs in YAML or JSON format. The specification acts as a contract between API providers and consumers.
AsyncAPI and AsynchApi
AsyncAPI is a specification designed for event-driven and message-based APIs. It documents how applications communicate through messages rather than HTTP requests.
The specification supports various messaging protocols like MQTT, AMQP, and Kafka. Developers use AsyncAPI to define message formats, channels, and connection details.
AsyncAPI tools generate documentation and code for both publishers and subscribers. This makes it simpler to build and maintain message-driven systems.
API Blueprints
API Blueprint is a markdown-based specification format. It uses simple syntax to describe API resources, actions, and parameters.
The format focuses on human readability while still being machine-processable. Developers can quickly write and update API documentation using familiar markdown syntax.
API Blueprint tools can generate interactive documentation, test cases, and mock servers. Many teams choose this format for its simplicity and ease of use.
Designing RESTful APIs
RESTful APIs enable applications to communicate through HTTP requests and responses. They follow key principles like resource-based design and stateless communication.
Resources and Methods
Resources form the foundation of REST APIs. Each resource needs a unique identifier (URI) that clients use to interact with it.
Common resource examples include users, products, or orders. The URI should clearly identify the resource: /users/123
or /products/456
.
REST APIs use standard HTTP methods to work with resources:
- GET - Retrieve data
- POST - Create new resources
- PUT - Update existing resources
- DELETE - Remove resources
HTTP Status Codes and Verbs
HTTP status codes tell clients what happened with their request:
- 2xx codes show success (200 OK, 201 Created)
- 4xx codes indicate client errors (400 Bad Request, 404 Not Found)
- 5xx codes mean server errors (500 Internal Server Error)
Each status code serves a specific purpose. Using the right code helps clients understand the response.
Endpoints and Collections
API endpoints represent specific resources or collections. Collection endpoints like /users
handle groups of resources.
Single resource endpoints use identifiers: /users/123
. This pattern makes the API predictable and easy to use.
Keep endpoint names simple and descriptive. Use nouns for resources and avoid verbs in URLs.
Use query parameters for filtering and sorting: /products?category=electronics&sort=price
Advanced API Topics
API design at scale requires specific architectural patterns and performance optimizations to handle complex system requirements and heavy loads. These advanced approaches help create robust, maintainable, and high-performing APIs.
Microservices and Scalability
Microservices architecture breaks down large applications into small, independent services that communicate through APIs. Each service handles a specific business function and can scale independently.
Key benefits of microservices include:
- Isolated Deployment: Teams can update services without affecting others
- Independent Scaling: High-traffic services can scale up without wasting resources
- Technology Flexibility: Different services can use different programming languages
Services communicate through lightweight protocols like HTTP/REST or gRPC. Load balancers distribute traffic across multiple instances of each service to maintain performance under heavy loads.
Event-Driven Architectures
Event-driven APIs use a publish-subscribe model where services emit and react to events rather than making direct requests.
Message brokers like Apache Kafka or RabbitMQ handle event distribution between services. This creates loose coupling between components.
Benefits of event-driven design:
- Real-time Updates: Services react to changes immediately
- Better Fault Tolerance: Failed events can be replayed
- Improved Performance: Async processing reduces system load
Caching and Statelessness
Stateless APIs treat each request as independent, storing no client data between calls. This makes scaling easier since any server can handle any request.
Caching speeds up responses by storing frequent data:
- Client-side: Browsers cache responses
- CDN: Geographic distribution of cached content
- Server-side: In-memory stores like Redis
Cache invalidation strategies keep data fresh:
- Time-based expiration
- Manual purging
- Version tags
API Security Measures
Strong security measures protect APIs from unauthorized access and data breaches. Security strategies must include robust authentication, authorization protocols, and data protection.
Authentication and Authorization
Authentication verifies user identity through credentials like usernames and passwords. The process checks if users are who they claim to be before granting access.
Authorization determines what actions authenticated users can perform. Role-based access control (RBAC) assigns specific permissions to different user roles.
Regular security audits help identify gaps in authentication and authorization systems. These audits should check for weak passwords, expired credentials, and incorrect permission settings.
API Keys and OAuth 2.0
API keys act as unique identifiers for applications or users accessing the API. They track usage, enforce rate limits, and provide basic security.
OAuth 2.0 offers secure third-party access without sharing passwords. It uses tokens to grant temporary access to specific resources.
The flow includes:
- Client registration
- User authorization
- Token exchange
- Secure resource access
Security Features and JWTs
JSON Web Tokens (JWTs) securely transmit information between parties. They contain encoded data about the user and their permissions.
Key security features include:
- SSL/TLS encryption for data in transit
- Rate limiting to prevent abuse
- Input validation to block malicious data
- IP whitelisting for trusted sources
Regular security updates patch vulnerabilities and strengthen defenses. Monitoring systems should track unusual activity patterns and potential threats.
API Performance Optimization
Good API design requires careful attention to performance and scalability. Fast response times and reliable throughput help create positive experiences for API consumers.
Rate Limiting and Throttling
Rate limiting protects APIs from excessive use by restricting the number of requests a client can make in a specific time period. Common limits include requests per second, minute, or hour.
Key Rate Limiting Strategies:
- Token bucket algorithm allows short traffic bursts while maintaining long-term rate limits
- Fixed window counting tracks requests within defined time slots
- Sliding window tracking provides smoother request distribution
APIs should return clear rate limit information in response headers:
- X-RateLimit-Limit: Maximum requests allowed
- X-RateLimit-Remaining: Requests remaining
- X-RateLimit-Reset: Time until limit resets
Monitoring and Feedback
Regular performance monitoring helps identify issues before they impact users. Key metrics to track include response times, error rates, and resource usage.
Essential Monitoring Elements:
- Response time percentiles (p95, p99)
- Request volume and throughput
- CPU and memory utilization
- Database query performance
Real-time alerts should trigger when metrics exceed defined thresholds. This allows quick response to performance problems.
Collecting user feedback through developer forums and support channels provides valuable insight into real-world API performance needs.
API Documentation and Tools
Good API documentation and the right set of tools help developers build better integrations faster. Tools like Swagger and Apidog have transformed how teams create, test, and share API documentation.
Creating Comprehensive API Docs
API documentation needs clear explanations of endpoints, parameters, and response formats. Modern documentation tools generate interactive docs from OpenAPI specifications.
Key Documentation Elements:
- Request/response examples
- Authentication methods
- Error codes and handling
- Code snippets in multiple languages
- Getting started guides
Teams can use tools like Swagger UI to create docs that let developers test API calls directly in the browser. This hands-on approach speeds up the learning process.
Mock Servers and Testing
Mock servers simulate API responses during development. This lets front-end teams work without waiting for the back-end to be ready.
Popular Testing Features:
- Response simulation
- Network latency testing
- Error scenario testing
- Automated test cases
- Load testing capabilities
Tools like Apidog combine mocking and testing in one platform. This makes it easier to validate API behavior across different use cases.
API Tools for Developers
Modern API tools help with design, testing, and monitoring. Many platforms offer integrated solutions for the full API lifecycle.
Essential Developer Tools:
- API design editors
- Version control integration
- Code generation
- Debug tooling
- Performance monitoring
Teams can use these tools to catch issues early and maintain consistent API quality. Integration with existing development workflows helps adoption.
The best tools support collaboration between team members and make it simple to keep documentation up to date as APIs evolve.
Managing API Evolution
API changes require careful planning to maintain compatibility while adding new features. Breaking changes can disrupt client applications and business operations if not handled properly.
Versioning Strategies
API versions help teams introduce changes without disrupting existing clients. The URI path versioning method adds version numbers directly in URLs like /v1/users
. This makes versions explicit and easy to understand.
Header versioning uses custom HTTP headers to specify versions. This keeps URLs clean but requires more client configuration.
Content negotiation lets clients request specific versions through Accept headers. This follows REST principles and provides flexibility.
Version Numbering Options:
- Semantic versioning (2.1.0)
- Date-based (2025-02)
- Simple incrementing (v1, v2)
Maintaining API Contracts
API contracts define the expected behavior between providers and consumers. Written documentation and interface specifications form the basis of these contracts.
Teams should track all API changes in a changelog. Small updates can use backward-compatible additions rather than new versions.
Contract Best Practices:
- Document all endpoints and parameters
- Keep old versions available during transition periods
- Set clear deprecation timelines
- Test contract compliance automatically
- Communicate changes to API consumers early
Breaking changes require new versions. Non-breaking changes like adding optional fields can use the existing version.
API Design Best Practices
Good API design focuses on creating clear, efficient, and user-friendly interfaces. Proper design choices make APIs easier to use, maintain, and scale over time.
API-First vs Code-First Approach
The API-first approach starts with designing the API interface before writing any code. Teams create detailed API specifications and documentation upfront, which serves as a contract between different parts of the system.
API-first design lets teams work in parallel since the interface is defined early. Frontend developers can start building applications while backend teams implement the actual functionality.
The code-first method begins with the implementation and generates API documentation afterward. This approach works well for smaller projects or when rapid prototyping is needed.
Utilizing Design Patterns
Common API Design Patterns:
- Resource-based URLs for clear endpoint naming
- Consistent error handling across all endpoints
- Proper use of HTTP methods (GET, POST, PUT, DELETE)
- Standardized response formats
Versioning helps manage API changes without breaking existing client applications. Teams should choose between URL versioning (v1/users) or header-based versioning based on their needs.
Gathering User Feedback
Regular feedback from API consumers helps identify pain points and areas for improvement. Teams should set up multiple feedback channels like:
- Developer forums
- Issue tracking systems
- Usage analytics
- Direct user interviews
Testing new API features with a small group of users helps catch problems early. Teams can use beta programs or staged rollouts to gather real-world usage data.
API metrics track response times, error rates, and popular endpoints. This data guides future improvements and helps maintain API performance.