API Documentation Best Practices for Modern Development Teams
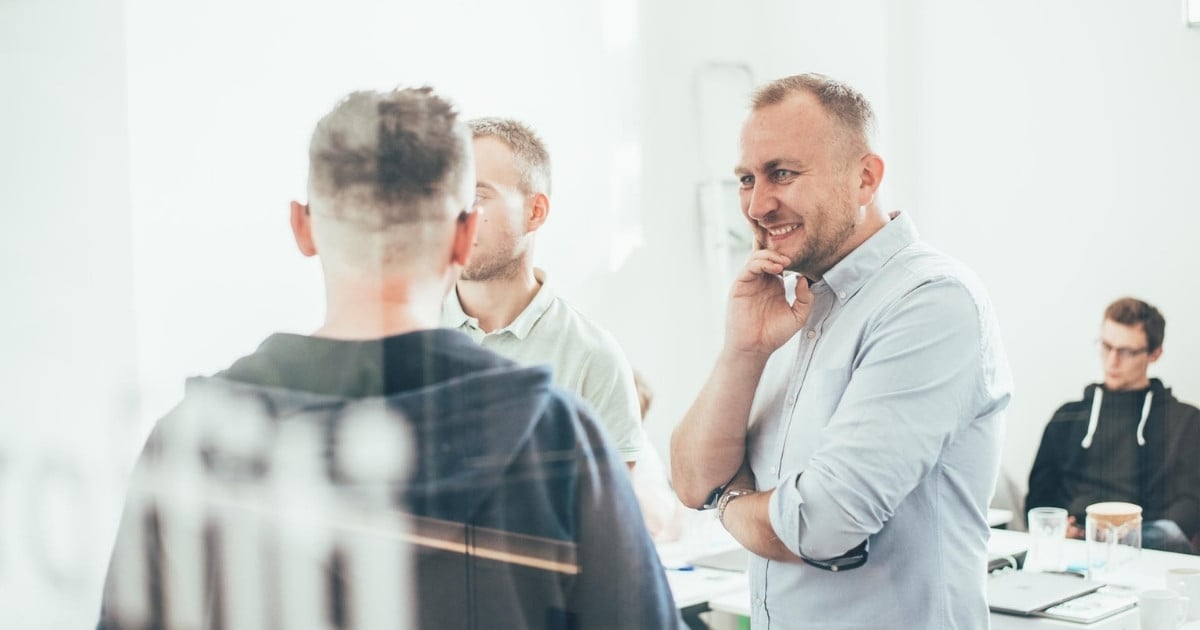
Writing API documentation requires careful attention to detail and a focus on user needs. Code examples, clear explanations, and proper formatting help developers quickly grasp the core concepts and start using the API. Tools like Swagger, Postman, and Document360 make it easier to create and maintain high-quality documentation.
Key Takeaways
- Clear and well-structured documentation speeds up integration and reduces developer frustration
- Code examples and detailed endpoint descriptions help developers understand API functionality
- Regular updates and proper maintenance ensure documentation stays accurate and useful
Getting Started
API documentation requires a clear path from initial setup through basic usage. The right tools and environment make development smooth and efficient.
Understanding APIs
An API (Application Programming Interface) lets different software systems communicate. APIs use standard protocols like HTTP to send and receive data between applications.
APIs follow specific rules for requests and responses. They define endpoints, methods, and data formats that developers must use correctly.
Most modern APIs use REST architecture and return JSON data. Learning these core concepts helps developers work effectively with any API.
Setting Up the Environment
Developers need specific tools to work with APIs:
- A code editor (VS Code, Sublime Text)
- API testing tool (Postman, Insomnia)
- Command line interface
- Required programming language and dependencies
- API keys or authentication credentials
Install these tools before starting development. Test the setup with a simple API request to confirm everything works.
Quick Start Guide
Start with a basic API request:
- Get API credentials from the provider
- Make a test request using Postman
- Check the response format
- Try different endpoints
Write simple code to connect to the API:
fetch('api-endpoint')
.then(response => response.json())
.then(data => console.log(data))
Test the connection with small requests first. Keep track of rate limits and API usage quotas.
API Authentication
API authentication lets systems verify who makes API calls and stops unauthorized access. Every API request needs proper credentials to work.
Authentication Methods
Basic authentication uses a username and password combination encoded in base64 format. Many APIs avoid this method because it's less secure than modern options.
OAuth 2.0 offers better security through tokens. It works well for apps that need to access user data from services like Google or Facebook.
Single Sign-On (SSO) lets users access multiple APIs with one login. This makes life easier for users while keeping systems secure.
API Keys
API keys are unique strings that identify who makes each API call. They work like a password for accessing API endpoints.
Most APIs give developers both test and live API keys. Test keys let developers try things out safely before going live.
Keys should stay private and never appear in public code. Many developers store API keys in secure environment variables.
Some APIs use different key types for different access levels. Read-only keys might allow viewing data, while full access keys let apps make changes.
Developers can often manage their API keys through a dashboard. This makes it easy to create new keys or remove old ones if they get exposed.
API Endpoints
API endpoints serve as specific URLs that enable access to resources and functionalities within an API. Each endpoint represents a distinct service point where clients can send requests and receive responses.
Resource Overview
API endpoints follow a structured URL pattern that maps to specific resources. For example, /users
might handle user data while /products
manages product information.
The base URL forms the foundation:
- Production:
https://api.example.com/v1/
- Development:
https://dev-api.example.com/v1/
Resource paths build on this base URL to create complete endpoints. Common URL patterns include:
- Single resource:
/users/{id}
- Collection:
/users
- Nested resources:
/users/{id}/orders
HTTP Methods
Each endpoint supports specific HTTP methods that define allowed actions on resources:
Common Methods:
GET
- Retrieves dataPOST
- Creates new resourcesPUT
- Updates entire resourcesPATCH
- Updates partial resourcesDELETE
- Removes resources
The API returns status codes to indicate request outcomes:
- 200-299: Success
- 400-499: Client errors
- 500-599: Server errors
Endpoints must specify required parameters and headers for proper request formatting. Authentication tokens often go in request headers for secure access.
Error Handling
APIs need clear and consistent error handling to help developers troubleshoot issues quickly. Error responses should include specific details about what went wrong and how to fix it.
Common Error Messages
Error messages in APIs follow a standard format that includes a message type, description, and error code. The message tells users what happened and why their request failed.
A good error message contains:
- Clear description of the problem
- Specific error code
- Suggested steps to resolve the issue
- Additional context when needed
Error responses should use simple language that developers can understand. Technical jargon makes errors harder to fix.
HTTP Status Codes
Status codes indicate if an API request succeeded or failed. The codes fall into five categories:
2xx - Success
- 200: OK
- 201: Created
- 204: No Content
4xx - Client Errors
- 400: Bad Request
- 401: Unauthorized
- 404: Not Found
5xx - Server Errors
- 500: Internal Server Error
- 503: Service Unavailable
Each status code helps developers know if the problem is on their end or the server's end. The code guides them toward the right solution.
API Reference
An API reference documents every endpoint, method, parameter and data type needed to make successful API calls. It serves as a detailed technical guide for developers implementing the API.
Request and Response Examples
Code samples show exactly how to structure API requests and handle responses. Each endpoint needs clear examples of both successful calls and error scenarios.
Request examples should display proper parameter formatting and authentication headers. Here's a basic example:
GET /api/v1/users
Authorization: Bearer abc123token
Response examples must show the returned JSON structure and status codes:
{
"users": [
{
"id": 1,
"name": "John Smith"
}
]
}
Data Types
Data types define the format of information sent to and received from the API. Common types include strings, numbers, booleans, and objects.
Parameters need specific type definitions and constraints. For example:
user_id
: Integer, requiredemail
: String, valid email formatis_active
: Boolean, optional
Arrays and nested objects require clear structure documentation:
{
"order": {
"items": [
{
"product_id": "Integer",
"quantity": "Integer"
}
]
}
}
Examples and Tutorials
Clear examples and practical tutorials help developers learn APIs faster and implement them correctly. Code samples paired with step-by-step guides reduce integration time and prevent common mistakes.
Sample Applications
Leading API providers offer complete sample applications that demonstrate real-world usage. Twilio provides working examples for SMS and voice features, while Stripe shows payment processing implementations.
Code repositories on GitHub let developers download and run functional demo apps. These samples cover authentication, data handling, and error management.
Many samples include both basic and advanced use cases. Simple examples demonstrate core features, while complex ones showcase API capabilities like webhooks and event handling.
Integration Guides
Step-by-step tutorials break down complex integration tasks into manageable pieces. Each guide focuses on a specific goal like sending messages or processing payments.
The best tutorials include:
- Code snippets in multiple programming languages
- Screenshots of key steps and configuration screens
- Common pitfalls and troubleshooting tips
- Sample responses and error messages
Popular APIs provide quickstart guides for different platforms. These help developers set up authentication, make test API calls, and validate their implementation.
Guides often link to related reference docs and support resources. This creates a complete learning path from basics to advanced usage.
Software Development Kits (SDKs)
Software Development Kits package essential tools, libraries, and resources that developers need to build applications for specific platforms. SDKs streamline development by providing pre-built code and functionality.
SDK Languages
SDKs are available for many popular programming languages like Python, Java, JavaScript, and C++. Each SDK includes language-specific libraries and tools suited to that environment.
Developers can choose SDKs that match their preferred programming language and development needs. Popular mobile SDKs support Swift for iOS and Kotlin for Android development.
Code libraries within SDKs contain pre-written functions and classes that handle common tasks. These save time by eliminating the need to write basic functionality from scratch.
Installation and Configuration
Most SDKs use package managers for easy installation. For example, Python SDKs install through pip, while JavaScript SDKs use npm.
The basic setup process includes:
- Installing the SDK package
- Adding authentication credentials
- Importing required libraries
- Configuring basic settings
SDK documentation provides step-by-step guides for proper installation. These guides include code examples showing how to initialize the SDK and verify it works correctly.
Many SDKs offer quick-start templates that help developers begin coding right away. These templates demonstrate best practices for SDK configuration and usage.
Specification and Tools
API specifications provide the foundation for creating clear and consistent documentation. Modern tools help developers generate and maintain high-quality API documentation automatically.
The OpenAPI Specification
OpenAPI serves as the industry standard for describing RESTful APIs. This specification defines a standard format for documenting API endpoints, parameters, responses, and authentication methods.
The specification uses YAML or JSON format to structure API information. This makes it both human-readable and machine-parsable.
Developers can write the specification once and use it to generate documentation, client libraries, and server stubs automatically. This approach saves time and reduces errors.
Key benefits of OpenAPI include:
- Consistency across documentation
- Machine-readable format
- Language-agnostic implementation
- Tool compatibility with many platforms
Using Swagger and SwaggerHub
Swagger offers a suite of tools built around the OpenAPI specification. These tools help teams design, build, and document APIs efficiently.
SwaggerHub provides a centralized platform for API development teams. It includes features like:
- Real-time collaboration
- Version control
- Auto-generated documentation
- Interactive API testing
Teams can create and edit API specifications directly in SwaggerHub's editor. The platform automatically validates changes and updates documentation in real-time.
The Swagger UI tool creates interactive documentation that lets developers test API endpoints directly from their web browser. This hands-on approach makes it easier to understand how the API works.
Best Practices
Clear and effective API documentation requires careful attention to both the writing style and document structure. Teams should follow established guidelines to create documentation that helps developers succeed.
Writing Documentation
Technical writers must use simple, direct language that developers can understand quickly. Short sentences and basic terminology work better than complex explanations.
API examples should cover common use cases and include both requests and responses. Code samples need proper formatting and syntax highlighting.
Writers should explain error messages and status codes clearly. Each error needs a description of why it occurs and how to fix it.
Documentation must stay current with API changes. Regular reviews help catch outdated information before it causes problems.
Documentation Structure
The documentation needs a logical flow from basic concepts to advanced features. A clear table of contents helps developers find information fast.
Each endpoint requires detailed descriptions of:
- Authentication requirements
- Request parameters
- Response formats
- Rate limits
- Resource URLs
Break long procedures into numbered steps. Use bullet points for lists of features or requirements.
Add a search function to help developers locate specific topics. Include links between related sections to improve navigation.
Consider adding a "Getting Started" guide for new users. This gives developers a quick path to making their first API call.
Advanced Features
API documentation requires specific technical capabilities to manage usage and track changes effectively. These features help developers control access and stay updated on modifications.
Rate Limiting
Rate limiting protects APIs from overuse by setting specific request thresholds. Most APIs implement limits based on time intervals, such as 1,000 requests per hour or 100 calls per minute.
Developers need clear documentation about these limits, including quota reset times and response headers that show remaining requests. The documentation should explain rate limit error codes and how to handle them.
Common rate limiting patterns include:
- IP-based restrictions
- Authentication token quotas
- Tiered access levels
- Burst allowances
Versioning and Changelog
API versions help maintain compatibility while allowing for improvements. Version numbers typically follow semantic versioning (major.minor.patch) to indicate the scale of changes.
A well-maintained changelog tracks all API modifications, additions, and removals. Each entry should include:
- Date: When the change occurred
- Version: The affected API version
- Type: Bug fix, feature, or breaking change
- Description: Clear explanation of what changed
Release notes complement the changelog by providing migration guides and code examples for major updates. This helps developers plan their integration updates.
Breaking changes need special attention in documentation, with advance notice given to API consumers. A deprecation schedule gives developers time to adapt their code.
Additional Resources
API documentation benefits from a variety of tools, communities, and support options that help developers create better documentation and get answers to their questions.
External Tools and Libraries
Postman provides a powerful platform for API testing and documentation generation. The tool allows teams to create mock servers, debug APIs, and generate detailed documentation automatically.
Stripe and PayPal offer excellent documentation templates that serve as industry benchmarks. Their tools help create interactive API references and sample code snippets.
Technical writers can use specialized tools like Swagger UI to create OpenAPI documentation. These tools generate clean, organized documentation with built-in navigation and search features.
Community and Support
Stack Overflow hosts a large community of developers who share solutions to API documentation challenges. Users can find answers about documentation best practices and implementation details.
Developer forums maintained by Twilio and other API providers offer direct support. These spaces let teams discuss common use cases and documentation improvements.
API documentation groups on LinkedIn and Discord connect technical writers with experienced professionals. Members share tips about software development documentation and terms of use creation.
GitHub repositories contain open-source documentation tools and templates. Teams can collaborate, share feedback, and improve their documentation through version control.