Java Concurrency: Essential Techniques for Efficient Multithreading
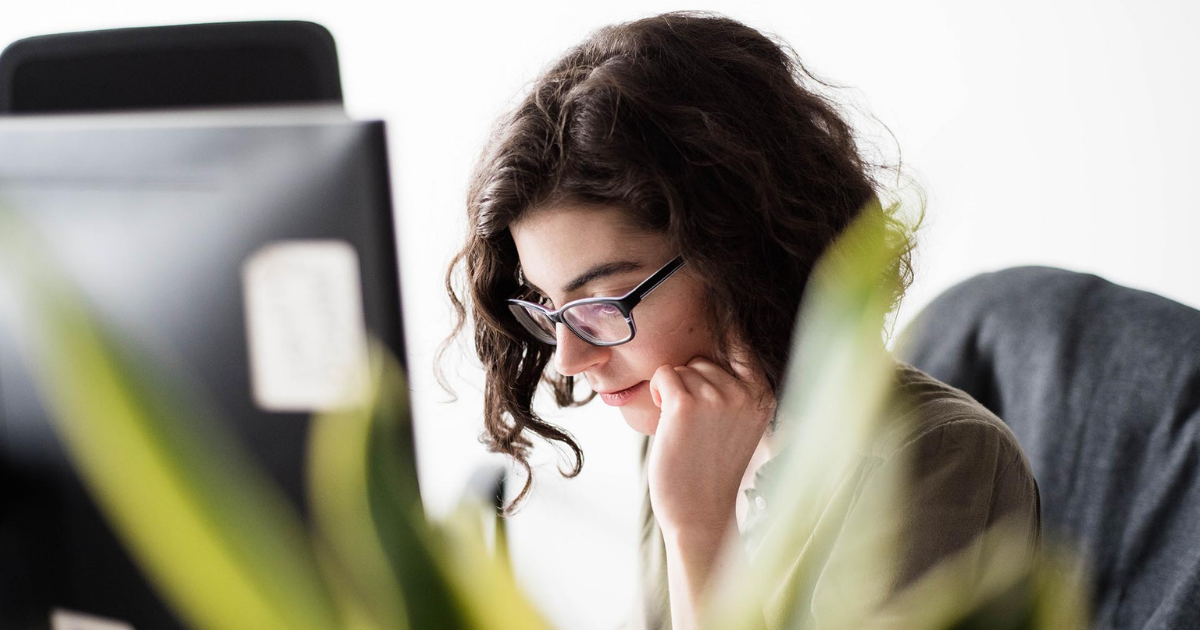
Java provides built-in support for concurrent programming through its language features and class libraries.
The Java platform offers various tools for managing concurrent tasks. These include basic thread creation, synchronization mechanisms, and high-level APIs in the java.util.concurrent package.
Developers can use these to build robust, scalable applications that take advantage of modern multi-core processors.
Learning Java concurrency is essential for writing efficient programs. It helps solve complex problems and enhances application performance. By mastering concurrency concepts, programmers can create software that runs faster and uses system resources more effectively.
Key Takeaways
- Java concurrency allows programs to perform multiple tasks at the same time
- The Java platform provides built-in support and tools for concurrent programming
- Understanding concurrency concepts helps create faster and more efficient applications
Understanding Java Concurrency
Java concurrency enables programs to perform multiple tasks simultaneously. It improves efficiency and responsiveness in applications. Proper use of concurrency features is key to building robust, high-performance Java systems.
Core Concepts of Concurrency
Threads are the basic units of concurrency in Java. A thread represents an independent path of execution within a program.
Multiple threads can run at the same time, sharing resources and memory.
Java provides built-in support for creating and managing threads. The Thread class and Runnable interface are fundamental tools for working with threads.
Synchronization is crucial in concurrent programming. It helps prevent race conditions, where multiple threads access shared data simultaneously.
Java offers synchronized methods and blocks to coordinate thread access to critical sections of code.
The java.util.concurrent package provides high-level concurrency utilities. These include thread pools, locks, and atomic variables. These tools simplify complex concurrent programming tasks.
Challenges in Concurrent Programming
Deadlocks occur when two or more threads are unable to proceed because each is waiting for the other to release a resource. Proper resource management and lock ordering can help avoid deadlocks.
Race conditions happen when the outcome of a program depends on the timing of thread execution. These bugs can be hard to reproduce and fix. Careful synchronization is needed to prevent race conditions.
Starvation is another issue in concurrent systems. It happens when a thread is unable to gain regular access to shared resources. This can lead to poor performance or unresponsiveness.
Shared data access is a common challenge. Multiple threads trying to read or write the same data can cause inconsistencies. Proper synchronization and use of thread-safe data structures are essential.
Concurrency Vs. Parallelism
Concurrency and parallelism are related but distinct concepts. Concurrency is about dealing with multiple tasks at once. Parallelism is about executing multiple tasks simultaneously.
Concurrency focuses on managing and coordinating different tasks. It can be achieved even on a single-core processor through time-slicing.
Parallelism requires multiple processors or cores to truly execute tasks at the same time.
Java supports both concurrency and parallelism. The Fork/Join framework, introduced in Java 7, is designed for parallel processing. It helps divide tasks into smaller subtasks that can be processed in parallel.
Structured concurrency is a newer approach in Java. It aims to simplify concurrent programming by providing better control over the lifecycle of tasks and subtasks. This can lead to more maintainable and less error-prone concurrent code.
Java Threads and Runnable Interface
Java offers two main ways to create and manage threads for concurrent programming. These approaches allow developers to run multiple tasks at the same time and make efficient use of system resources.
Creating Threads
Java provides two methods to create threads. The first is by extending the Thread class. This approach involves creating a new class that inherits from Thread and overriding its run() method. Here's a simple example:
class MyThread extends Thread {
public void run() {
System.out.println("Thread is running");
}
}
MyThread thread = new MyThread();
thread.start();
The second method is by implementing the Runnable interface. This way is often seen as more flexible:
class MyRunnable implements Runnable {
public void run() {
System.out.println("Thread is running");
}
}
Thread thread = new Thread(new MyRunnable());
thread.start();
Both methods achieve the same result but have different uses depending on the situation.
Thread Class Vs. Runnable Interface
The choice between extending Thread and implementing Runnable depends on design needs. Extending Thread can be limiting since Java doesn't support multiple inheritance. This means a class that extends Thread can't extend any other class.
Implementing Runnable is more flexible. It allows a class to implement other interfaces or extend other classes. This approach also promotes better separation between the task logic and the thread behavior.
Runnable is often seen as a better practice in object-oriented design. It lets developers reuse the same task with different thread objects or even thread pools.
Thread Management and Lifecycle
Thread management involves controlling the state and behavior of threads. Java threads have several states: New, Runnable, Running, Blocked, Waiting, and Terminated.
Developers can use methods like start(), sleep(), and join() to manage thread lifecycle. The start() method begins thread execution, sleep() pauses a thread for a set time, and join() makes one thread wait for another to finish.
Thread priorities can be set using setPriority() method. Priorities range from 1 (lowest) to 10 (highest).
Proper thread management is crucial for writing efficient concurrent programs. It helps avoid issues like deadlocks and race conditions.
Java also offers advanced concurrency utilities in the java.util.concurrent package. These tools provide higher-level threading concepts for more complex applications.
Synchronization and Locks
Java offers tools to manage shared data in multi-threaded programs. These tools help prevent errors and keep data safe when many threads work at once.
Synchronized Keyword and Blocks
The synchronized keyword is a basic way to control thread access. It works with methods or blocks of code. When a thread enters a synchronized area, it gets a lock. Other threads must wait until the lock is free.
Synchronized methods are easy to use. Just add the keyword to the method:
public synchronized void updateCounter() {
counter++;
}
Synchronized blocks target specific objects. They're more precise:
synchronized(this) {
// Code here is protected
}
The synchronized approach uses intrinsic locks. These locks are tied to objects in Java.
Lock Interface and Classes
The java.util.concurrent.locks package offers more advanced locking options. These locks give programmers more control than synchronized.
The main interface is Lock. It has methods like lock() and unlock(). Here's a basic example:
Lock myLock = new ReentrantLock();
myLock.lock();
try {
// Protected code here
} finally {
myLock.unlock();
}
Locks must be manually released. The try-finally block ensures this happens.
Other useful classes include ReadWriteLock and StampedLock. These allow for more complex locking patterns.
ReentrantLock
ReentrantLock is a popular Lock implementation. It's called "reentrant" because a thread can lock it multiple times.
Key features of ReentrantLock include:
- Fairness: You can make threads wait in order.
- Timed lock attempts: Try to get a lock, but give up after a set time.
- Interruptible locking: A thread can be interrupted while waiting for a lock.
Here's how to use a fair ReentrantLock:
ReentrantLock fairLock = new ReentrantLock(true);
if (fairLock.tryLock(5, TimeUnit.SECONDS)) {
try {
// Work with the protected resource
} finally {
fairLock.unlock();
}
}
This code tries to get the lock for 5 seconds. If it can't, it moves on.
Java Concurrency Utilities
Java provides powerful tools for building concurrent applications. These utilities simplify complex threading tasks and improve performance.
The Executors Framework
The Executors framework manages thread creation and execution. It offers thread pools that reuse threads, reducing overhead.
ExecutorService is a key interface in this framework. It lets you submit tasks for execution without manually creating threads.
Thread pools are central to the Executors framework. They maintain a set of reusable threads for running tasks. This saves time and resources compared to creating new threads for each task.
Common thread pool types include:
- Fixed thread pool
- Cached thread pool
- Scheduled thread pool
To create a fixed thread pool with 5 threads:
ExecutorService executor = Executors.newFixedThreadPool(5);
Concurrent Collections
Java offers thread-safe collections designed for concurrent access. These collections handle synchronization internally, improving performance and ease of use.
ConcurrentHashMap is a popular concurrent collection. It allows multiple threads to read and write simultaneously without external locking.
BlockingQueue is another useful concurrent collection. It supports operations that wait for the queue to become non-empty when retrieving an element, and wait for space to become available in the queue when storing an element.
Example using a BlockingQueue:
BlockingQueue<String> queue = new LinkedBlockingQueue<>();
queue.put("Task 1");
String task = queue.take();
Additional Synchronizers
Java provides several synchronization utilities beyond basic locks and semaphores. These tools help coordinate actions between threads.
CountDownLatch lets a thread wait until other threads complete a set of operations. It's useful for starting multiple threads at once or waiting for multiple threads to finish.
Semaphore controls access to a shared resource through a counter. It can limit the number of threads accessing a resource at once.
ThreadLocal allows each thread to have its own copy of a variable. This is useful for maintaining thread-specific state without synchronization.
Example using a CountDownLatch:
CountDownLatch latch = new CountDownLatch(3);
// Start 3 threads that call latch.countDown() when done
latch.await(); // Wait for all 3 threads to finish
Atomic Variables and Classes
Atomic variables and classes provide thread-safe operations without using locks. They help solve concurrency issues in Java programs.
Understanding Atomic Operations
Atomic operations happen all at once without interruption. They are key for thread safety in multi-threaded programs. Java's java.util.concurrent.atomic package has classes for atomic variables.
AtomicInteger, AtomicLong, and AtomicBoolean are common atomic classes. They allow safe updates to int, long, and boolean values across threads.
These classes use special CPU instructions for thread-safe operations. This makes them faster than using synchronized code in many cases.
Using Atomic Classes for Safe Publication
Atomic classes ensure safe publication of shared data between threads. They prevent race conditions and provide consistent views of variables.
AtomicReference is useful for sharing object references safely. It allows atomic updates to references, avoiding issues with partially constructed objects.
Atomic classes have methods like get(), set(), and compareAndSet(). These methods let threads read and update values safely.
Here's an example of using AtomicInteger:
AtomicInteger count = new AtomicInteger(0);
count.incrementAndGet(); // Safely adds 1
int value = count.get(); // Gets current value
Atomic variables work well for simple shared state. For more complex scenarios, other concurrency tools may be needed.
Advanced Concurrency in Java
Java offers powerful tools for building efficient concurrent applications. These advanced features help developers create scalable programs that can handle complex threading scenarios.
Multithreaded Applications and Scalability
Multithreaded applications in Java can greatly improve performance and responsiveness. They allow multiple tasks to run simultaneously, making better use of system resources.
Java provides the Executor framework for managing thread pools. This helps avoid the overhead of creating new threads for every task.
Thread pools can be easily scaled up or down based on system load. This flexibility is key for building applications that can handle varying workloads.
Proper synchronization is crucial in multithreaded programs. Java offers synchronized blocks and the synchronized keyword to protect shared resources from concurrent access.
Fork/Join Framework
The Fork/Join framework is designed for divide-and-conquer algorithms. It's especially useful for recursive tasks that can be broken down into smaller subtasks.
This framework uses a work-stealing algorithm. Idle threads can take tasks from busy ones, balancing the workload across all available processors.
Key classes in this framework include ForkJoinPool and RecursiveTask. ForkJoinPool manages the thread pool, while RecursiveTask represents a task that can be split into smaller pieces.
Using Fork/Join can lead to significant speed improvements for certain types of problems. It's particularly effective for CPU-intensive tasks that can be parallelized.
Virtual Threads (Project Loom)
Virtual threads are a new feature in Java, introduced as part of Project Loom. They aim to simplify concurrent programming and improve application scalability.
Unlike platform threads, virtual threads are very lightweight. Millions of them can be created without exhausting system resources.
Virtual threads are managed by the Java runtime, not the operating system. This allows for more efficient scheduling and context switching.
They use a continuation-based approach, which can greatly reduce the complexity of asynchronous code. This makes it easier to write and maintain concurrent applications.
Error Handling and Best Practices
Java concurrency requires careful error handling and following best practices. This helps create robust, efficient code that avoids common pitfalls.
Handling InterruptedException
InterruptedException is a checked exception in Java. It signals that a thread was interrupted while waiting or sleeping. Proper handling is key for responsive concurrent code.
Don't ignore InterruptedException. Catch and handle it explicitly.
One option is to re-interrupt the thread:
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
Another approach is to propagate the exception:
public void doWork() throws InterruptedException {
// Method code here
}
This lets the caller decide how to handle the interruption.
Concurrency Best Practices
Use high-level concurrency utilities from java.util.concurrent. These tools simplify thread management and synchronization.
Prefer immutable objects. They are thread-safe by design.
When mutable state is needed, use proper synchronization.
Avoid using Thread.stop(). It's unsafe and can lead to data corruption. Instead, use a shared boolean flag to signal thread termination:
private volatile boolean running = true;
public void run() {
while (running) {
// Thread logic here
}
}
public void stopThread() {
running = false;
}
Minimize lock scope. Hold locks for the shortest time possible. This reduces contention and improves performance.
Use thread pools via ExecutorService for better resource management. It helps control the number of active threads:
ExecutorService executor = Executors.newFixedThreadPool(5);
executor.submit(new MyTask());
Test concurrent code thoroughly. Use tools like stress tests and race detectors to find hidden bugs.
Performance Considerations
Optimizing concurrent Java applications requires careful attention to performance. Proper benchmarking and profiling help identify bottlenecks, while techniques to improve responsiveness and throughput enhance the user experience.
Benchmarking and Profiling Concurrent Applications
Benchmarking tools measure the speed and efficiency of concurrent code.
JMH (Java Microbenchmark Harness) is a popular choice for testing small code snippets. It helps compare different concurrency approaches.
Profilers like VisualVM and JProfiler analyze running applications. They show CPU usage, memory allocation, and thread behavior. This data pinpoints performance issues in concurrent code.
Thread dump analysis reveals deadlocks and thread contention. It's useful for finding synchronization problems that slow down applications.
Improving Responsiveness and Throughput
Thread pools boost performance by reusing threads. This cuts down on thread creation overhead. The ExecutorService interface simplifies thread pool management.
Proper synchronization is key.
Using atomic variables and concurrent collections can increase throughput. They often perform better than synchronized blocks.
Batching tasks can improve efficiency. Instead of processing items one by one, group them. This reduces context switching and improves overall throughput.
Caching frequently accessed data minimizes thread contention. Local caching in thread-local storage can speed up concurrent operations.
Asynchronous programming models, like CompletableFuture, can enhance responsiveness. They allow non-blocking operations, keeping the application responsive during long-running tasks.
Design Patterns for Concurrency
Design patterns help solve common problems in concurrent Java programming. They provide tested solutions for managing threads, synchronization, and data sharing between components.
Common Concurrent Design Patterns
The Producer-Consumer pattern separates data creation from processing. Producers add items to a shared buffer, while consumers remove and use them. This allows for efficient task distribution.
The Thread Pool pattern reuses a fixed set of threads to execute tasks. It reduces overhead from creating new threads for each task. Thread pools are ideal for applications that process many short-lived operations.
The Read-Write Lock pattern allows multiple threads to read shared data concurrently. It restricts write access to one thread at a time. This improves performance for read-heavy workloads.
Patterns for Thread Safety and Synchronization
The Immutable Object pattern creates objects that can't be changed after construction. This eliminates the need for synchronization when sharing data between threads.
The Monitor Object pattern uses synchronized methods to control access to an object. Only one thread can execute a synchronized method at a time. This ensures data consistency.
The Double-Checked Locking pattern reduces synchronization overhead in singleton creation. It checks a lock twice before creating an instance. This pattern must be used carefully to avoid subtle bugs.
Concurrency in Practice
Concurrency plays a key role in many real-world Java applications. It allows programs to handle multiple tasks at once and make efficient use of system resources.
Real-World Use Cases
Web servers use concurrency to handle many user requests at the same time. Each request runs in its own thread, letting the server respond to multiple users quickly.
Financial systems rely on concurrency for fast trading and real-time data processing. Concurrent threads can update stock prices, execute trades, and analyze market trends in parallel.
Mobile apps leverage concurrency to keep the user interface responsive. Background tasks like network calls and data processing run on separate threads without freezing the UI.
Case Studies of Concurrency Problems
A major e-commerce site faced slowdowns during peak shopping times. The issue stemmed from thread contention when accessing a shared inventory database. They fixed it by using read-write locks and connection pooling.
A social media app crashed due to race conditions in its notification system. Multiple threads tried to update the same user data simultaneously. The fix involved using atomic operations and synchronized blocks.
A banking app had inconsistent account balances from concurrent transactions. They solved this by implementing database transactions and optimistic locking to maintain data integrity.