Java Data Structures: Essential Concepts for Efficient Programming
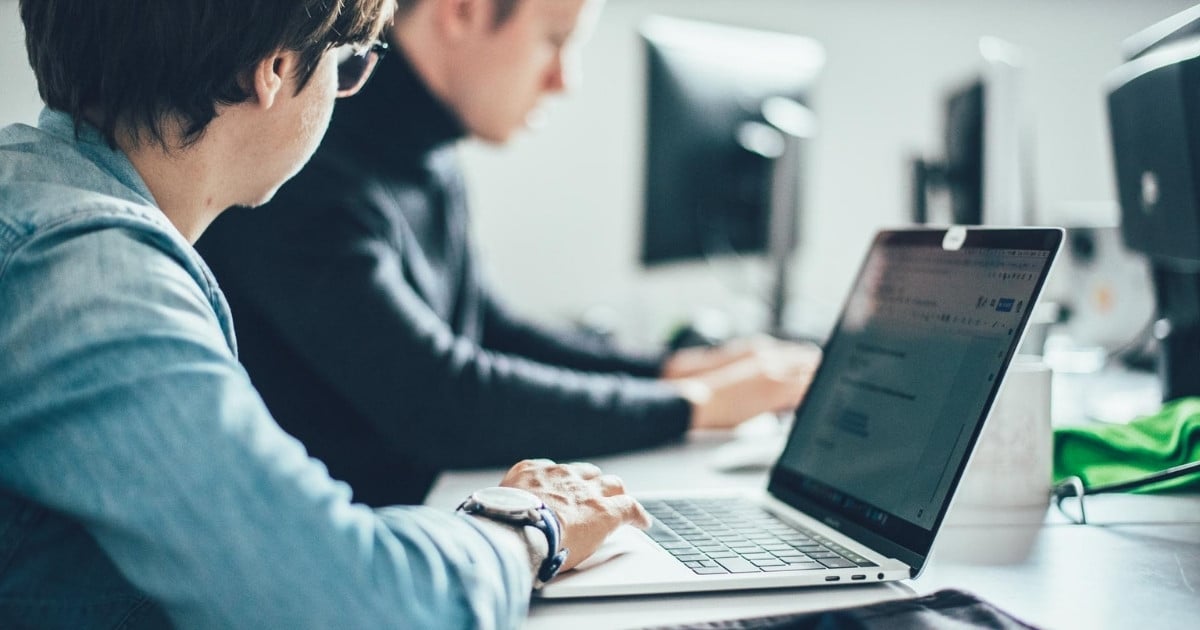
These structures include arrays, lists, stacks, queues, and trees. Each type has its own strengths and uses. For example, arrays are great for storing fixed-size collections of items. Lists work well for dynamic collections that can grow or shrink. Stacks and queues are useful for managing data in specific orders.
Learning about Java data structures is key for writing good programs. It helps coders pick the right tool for each task. This can make programs run faster and use less memory. It also makes code easier to read and maintain.
Key Takeaways
- Java provides various data structures for efficient data organization and management
- Different structures are suited for specific tasks and data types
- Understanding data structures improves code performance and readability
Understanding Data Structures in Java
Data structures in Java help organize and store information. They come in different types and shapes to fit various needs. Let's explore the main kinds and why they matter for Java programming.
Primitive vs. Non-Primitive Data Structures
Primitive data structures in Java are simple and built-in. They include int, char, float, and boolean. These store single values and take up a fixed amount of memory.
Non-primitive data structures are more complex. They can hold multiple values or objects. Arrays, classes, and interfaces fall into this group. These structures are flexible and can grow or shrink as needed.
Java offers ready-made non-primitive structures like ArrayList and LinkedList. Developers can also create custom ones to fit specific needs.
Linear vs. Non-Linear Data Structures
Linear data structures store items in a row. Each element links to the next one in line. Arrays and linked lists are common examples.
Arrays keep items in order and allow quick access. Linked lists make it easy to add or remove items anywhere in the list.
Non-linear structures branch out like trees or networks. They don't follow a straight line. Trees and graphs belong to this group.
These structures help solve complex problems. They're great for tasks like searching and sorting large amounts of data.
The Role of Data Structures in Java Programming
Data structures are key to writing good Java code. They help programs run faster and use less memory. Picking the right structure can make a big difference in how well a program works.
For instance, HashMaps are great for quick lookups. ArrayLists work well for lists that change size often. Knowing when to use each type is an important skill for Java developers.
Data structures also make code cleaner and easier to understand. They provide clear ways to organize information. This helps teams work together on big projects.
Learning about data structures is crucial for solving real-world problems with Java. It's a core part of becoming a skilled Java programmer.
Arrays and Their Implementation
Arrays store multiple values of the same type in a single variable. They are key building blocks in Java programming. Arrays can be one-dimensional or multi-dimensional and have many uses.
Single-Dimensional Arrays
Single-dimensional arrays hold a list of items. To make one, specify the data type and size:
int[] numbers = new int[5];
This creates an array that can hold 5 integers. You can add values like this:
numbers[0] = 10;
numbers[1] = 20;
Arrays start at index 0. To get a value, use its index:
int firstNumber = numbers[0]; // Gets 10
You can also make arrays with values right away:
String[] fruits = {"apple", "banana", "orange"};
Multi-Dimensional Arrays
Multi-dimensional arrays are arrays of arrays. They can represent tables or matrices. To make a 2D array:
int[][] grid = new int[3][3];
This creates a 3x3 grid. Add values like this:
grid[0][0] = 1;
grid[1][1] = 5;
You can also initialize 2D arrays with values:
int[][] matrix = ;
To get a value, use two indexes:
int center = matrix[1][1]; // Gets 5
Array to Matrix Conversion
Arrays can be turned into matrices for math operations. Here's how to convert a 1D array to a 2D matrix:
int[] array = {1, 2, 3, 4, 5, 6};
int rows = 2;
int cols = 3;
int[][] matrix = new int[rows][cols];
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
matrix[i][j] = array[i * cols + j];
}
}
This code turns a 1D array into a 2x3 matrix. It's useful for image processing and data analysis. The reverse process can change a matrix back to an array if needed.
Essential Java Collections Framework
The Java Collections Framework provides key data structures for storing and managing groups of objects. It offers flexible and efficient ways to work with collections of data.
ArrayList and LinkedList
ArrayList is a dynamic array implementation. It allows fast random access and is good for scenarios where you need quick retrieval by index. ArrayList grows automatically as elements are added.
LinkedList uses a doubly-linked list structure. It excels at insertions and deletions, especially at the beginning or end of the list. LinkedList is ideal for applications that frequently add or remove elements.
Both ArrayList and LinkedList implement the List interface. This means they share common methods for adding, removing, and accessing elements. The choice between them depends on the specific needs of your program.
Vector and Stack Implementations
Vector is similar to ArrayList but is synchronized. This makes it thread-safe for use in multi-threaded programs. Vector automatically grows as needed when elements are added.
Stack extends Vector and implements a last-in-first-out (LIFO) stack. It provides push and pop operations for adding and removing elements from the top of the stack.
Both Vector and Stack are considered legacy classes. For most new code, ArrayList or LinkedList are preferred over Vector. For stack operations, developers often use ArrayDeque instead of Stack.
These classes offer important functionality but have some drawbacks in performance compared to their modern counterparts.
Advanced Data Structures
Java offers powerful tools for managing complex data. These structures help programmers solve tricky problems and work with large amounts of information.
Stacks in Detail
A stack is like a pile of plates. You can only add or remove items from the top. In Java, the Stack class lets you use this structure.
Stacks follow the "last in, first out" (LIFO) rule. This means the last item you put on the stack is the first one you can take off.
Stacks are great for tasks like undoing actions or keeping track of function calls. They have methods like push() to add items and pop() to remove them.
Understanding Queues and Priority Queues
Queues work like a line at a store. The first person in line is the first to be helped. This is called "first in, first out" (FIFO).
Java has a Queue interface with classes like LinkedList that can act as queues. These are good for tasks that need to process items in order.
Priority queues are special. They let some items "cut in line" based on their importance. Java's PriorityQueue class does this automatically.
Trees and Tree-Based Structures
Trees are like upside-down family trees in computer science. They start with a "root" and branch out into "nodes."
Binary trees are common. Each node can have up to two "children." They're useful for searching and sorting data quickly.
Java doesn't have a built-in tree class, but you can make your own. Trees help with tasks like organizing file systems or making decision algorithms.
Graphs and Graph-Based Algorithms
Graphs are sets of points (vertices) connected by lines (edges). They can show relationships between many items.
In Java, you can make graphs using classes and lists. There's no standard graph class, so programmers often create their own.
Graphs solve real-world problems like finding the best route on a map or suggesting friends on social media. They use special math to find paths and connections.
Linked List Concepts
Linked lists are key data structures in Java. They use nodes to store and link data in a sequence. Linked lists offer flexible ways to organize information.
Singly vs. Doubly Linked Lists
Singly linked lists have nodes that point to the next node. Each node holds data and a link to the following node. The last node points to null.
Doubly linked lists have nodes that point both forward and backward. Each node has data plus links to the next and previous nodes. This allows moving in both directions through the list.
Singly linked lists use less memory. They work well for forward-only operations. Doubly linked lists take more space but allow backward traversal. They're better for tasks needing two-way movement.
Implementing a Custom Linked List in Java
Creating a custom linked list in Java starts with a Node class. This class holds the data and link(s) to other nodes. A LinkedList class manages the list operations.
The LinkedList class keeps track of the head node. It has methods to add, remove, and find nodes. Common methods include:
- add() to insert new nodes
- remove() to delete nodes
- get() to retrieve data
- size() to count nodes
Here's a basic Node class structure:
class Node {
int data;
Node next;
Node(int d) {
data = d;
next = null;
}
}
This forms the building block of a singly linked list. For a doubly linked list, add a 'prev' field to link backward.
Binary Trees and Binary Search Trees
Binary trees and binary search trees are key data structures in Java programming. They offer efficient ways to organize and search data.
Basics of Binary Trees
A binary tree is a structure where each node has at most two children. These children are called the left child and right child. The topmost node is the root. Nodes with no children are leaves.
Binary trees can be used to represent hierarchical data. They're useful for expression parsing and decision-making processes.
There are different types of binary trees. A full binary tree has every node with either zero or two children. A complete binary tree fills all levels except maybe the last.
Binary Search Tree Operations
Binary search trees (BSTs) are special binary trees. In a BST, the left child's value is always less than its parent. The right child's value is always greater.
This property makes BSTs great for searching. To find a value, start at the root. If the value is less, go left. If greater, go right. Repeat until found or reach a leaf.
Inserting in a BST is similar. Compare with nodes as you go down. Place the new value where it fits the BST rule.
Deleting is trickier. If the node has no children, just remove it. With one child, replace the node with its child. With two children, find the next highest value and use it to replace the deleted node.
BSTs offer fast search, insert, and delete operations. They're widely used in computer science for efficient data management.
Utilizing Hash-Based Structures
Hash-based structures offer fast data access and storage. They use special functions to map keys to specific locations, enabling quick retrieval and manipulation of information.
Hashtable and Its Applications
A hashtable stores key-value pairs for speedy lookups. It uses a hash function to compute an index where a value is stored or retrieved. This allows for constant-time average performance for basic operations.
Java's HashMap class is a common hashtable implementation. It's useful for caching, counting word frequencies, and removing duplicates from data sets. Here's a simple example:
HashMap<String, Integer> wordCount = new HashMap<>();
wordCount.put("apple", 5);
wordCount.put("banana", 3);
int appleCount = wordCount.get("apple"); // Returns 5
Hashtables excel at tasks like spell-checking, where quick word lookups are crucial. They're also great for implementing caches to speed up repeated computations.
Working with Tries and Their Usage
A trie is a tree-like structure ideal for storing and searching strings. Each node in a trie represents a character, and paths from the root to leaf nodes form complete words.
Tries are excellent for prefix-based searches and autocomplete features. They use less space than hashtables for certain datasets and offer fast prefix matching. Here's a basic trie node structure:
class TrieNode {
HashMap<Character, TrieNode> children = new HashMap<>();
boolean isEndOfWord;
}
Tries shine in applications like autocomplete systems, IP routing tables, and word games. They allow for quick validation of word existence and prefix searches, making them valuable in text processing and search algorithms.
Heaps and Priority Queues
Heaps and priority queues are key data structures in Java for managing elements based on priority. They allow efficient access to the highest or lowest priority item.
Understanding Heaps
A heap is a special type of binary tree. It follows a specific order where parent nodes have higher (or lower) priority than their children. This structure allows quick access to the top priority element.
There are two main types of heaps:
- Max heap: The parent is always larger than its children.
- Min heap: The parent is always smaller than its children.
Heaps are often used to make priority queues work faster. They let us find and remove the most important item quickly.
Implementation of Priority Queues
A priority queue is a data structure that stores elements with assigned priorities. It can be built using different methods, but heaps are a popular choice.
Java provides a PriorityQueue class in the java.util package. By default, it acts as a min heap. This means the smallest element has the highest priority.
To use a PriorityQueue:
- Create an instance:
PriorityQueue<Integer> pq = new PriorityQueue<>();
- Add elements:
pq.add(5);
- Get the top element:
int top = pq.peek();
- Remove the top element:
int removed = pq.poll();
Custom ordering can be set by passing a Comparator to the PriorityQueue constructor. This allows for max heaps or other custom priority rules.
Sorting and Searching Algorithms
Java offers many ways to sort data and find items quickly. These tools help organize information and locate specific pieces efficiently.
Sorting Techniques in Java
Java provides several sorting methods for arrays and lists. The Arrays.sort() method uses quicksort for primitive types and mergesort for objects. It's fast and works well for most cases.
For more control, Java has the Collections.sort() method. This lets you define custom sorting rules.
Bubble sort is simple but slow for large datasets. It swaps adjacent items until the list is in order.
Insertion sort works well for small lists. It builds the final sorted array one item at a time.
Mergesort splits the data, sorts the parts, and combines them. It's stable and handles large datasets well.
Quicksort is often the fastest. It picks a pivot, puts smaller items before it and larger ones after, then sorts the sections.
Search Operations and Their Efficiency
Linear search checks each item in order. It's simple but slow for big lists.
Binary search is faster for sorted data. It repeatedly splits the list in half to find the target.
Java's Arrays.binarySearch() method uses binary search on sorted arrays. It's quick and easy to use.
For objects, you can use the Collections.binarySearch() method. This works on sorted lists.
Hash-based searches are very fast. They use a hash function to find items directly.
Java's HashMap class offers constant-time lookups. It's great for key-value pairs.
Tree-based searches balance speed and flexibility. They work well for sorted data that changes often.
Specialized Java Data Structures
Java offers unique data structures that cater to specific needs. These structures help programmers solve complex problems efficiently. Let's explore some of Java's specialized data structures.
The BitSet Class
BitSet is a special class in Java that stores bits. It uses an array of long integers to represent a set of bits. BitSet is useful for tasks that involve flags or boolean values.
BitSet can grow dynamically as needed. It starts with a default size of 64 bits. When more bits are needed, it expands automatically.
Some key operations with BitSet include:
- set(): Sets a bit to true
- clear(): Sets a bit to false
- get(): Checks if a bit is set
- and(), or(), xor(): Perform logical operations on BitSets
BitSet is memory-efficient for large sets of boolean values. It's often used in areas like data compression and error detection.
Enumeration Interface and Iteration
The Enumeration interface in Java provides a way to iterate through a collection of elements. It's an older interface, but still used in some parts of the Java API.
Enumeration has two main methods:
- hasMoreElements(): Checks if there are more elements
- nextElement(): Returns the next element
While Enumeration is still supported, the Iterator interface is now more common. Iterator offers similar functionality but adds the ability to remove elements.
For example, to use an Enumeration:
Vector<String> v = new Vector<>();
v.add("Apple");
v.add("Banana");
Enumeration<String> e = v.elements();
while (e.hasMoreElements()) {
System.out.println(e.nextElement());
}
Properties and Custom Data Structures
The Properties class is a specialized subclass of Hashtable. It's designed to store string key-value pairs. Properties are often used for configuration settings in Java applications.
Key features of Properties:
- Can be easily saved to or loaded from a file
- Supports default values
- Hierarchical structure (one Properties object can have another as its default)
Example usage:
Properties props = new Properties();
props.setProperty("username", "admin");
props.setProperty("password", "secret");
String user = props.getProperty("username");
Custom data structures can be created in Java to fit specific needs. These might include specialized trees, graphs, or other complex structures. Custom structures often extend or implement existing Java interfaces to ensure compatibility with standard Java methods.