Java Microservices: Essential Strategies for Scalable Application Architecture
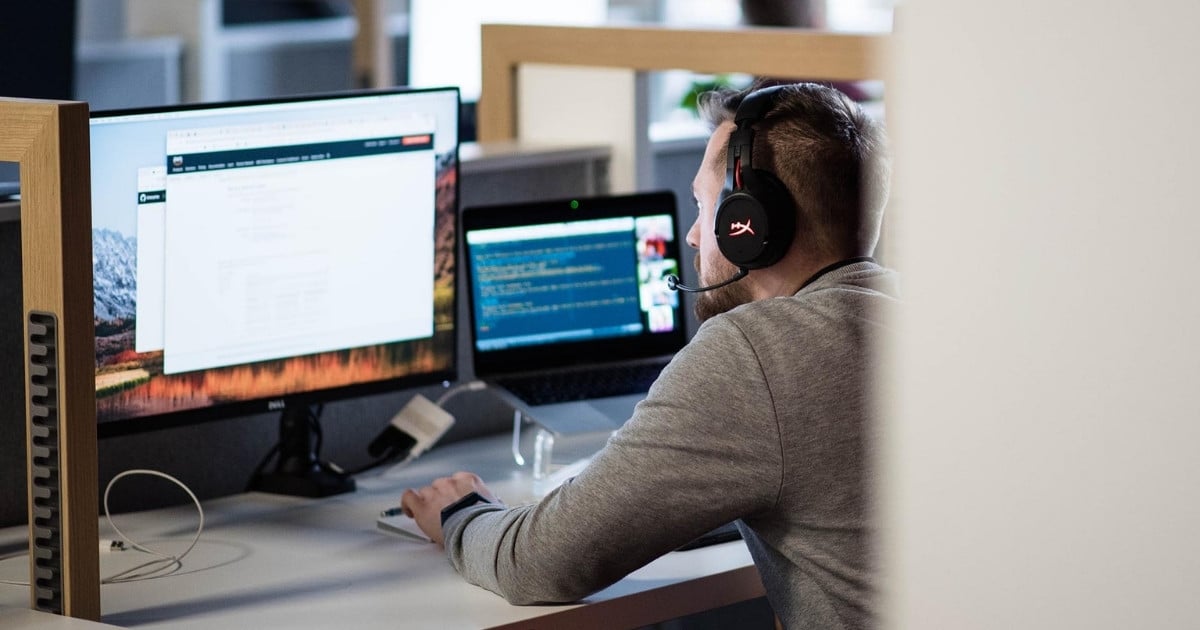
Java microservices offer flexibility and speed for modern applications. Developers can use tools like Spring Boot to create standalone services quickly. These services can communicate with each other, but they run independently. This means teams can work on different parts of an app without affecting the whole system.
Java's rich ecosystem supports microservices well. It provides libraries and frameworks that help with common tasks. These include service discovery, load balancing, and fault tolerance. Java's maturity also means there are many developers who can work on microservices projects.
Key Takeaways
- Java microservices break apps into small, independent parts for easier development and scaling
- Spring Boot and other Java tools simplify creating and managing microservices
- Java's ecosystem offers strong support for microservices with helpful libraries and frameworks
Understanding Microservices
Microservices are a modern approach to building software applications. They break down large systems into smaller, independent parts that work together. This design helps teams create flexible and scalable software more easily.
Microservices Basics
Microservices are small, focused software components. Each microservice handles a specific task or function within a larger application. These services run independently and communicate through APIs.
Microservices are built around business capabilities. For example, an e-commerce site might have separate services for user accounts, product catalogs, and order processing.
Teams can develop, deploy, and scale microservices separately. This allows for faster updates and easier maintenance. Microservices often use containers like Docker for consistent deployment across different environments.
Microservices vs Monolithic Architecture
Monolithic applications are built as a single, unified unit. All functions are managed and served in one place. This can make them simpler to develop initially, but harder to change over time.
Microservices split functions into separate services. This makes the system more flexible and easier to update. Teams can work on different services without affecting the whole application.
Monoliths can be faster to build at first, but microservices offer more benefits as applications grow. Microservices are easier to scale, as teams can add resources only where needed.
Advantages of Microservices
Microservices offer better scalability. Teams can scale individual services based on demand, rather than the entire application. This leads to more efficient resource use and cost savings.
They provide greater flexibility in technology choices. Different services can use different programming languages or databases that best fit their needs.
Microservices support faster development and deployment. Teams can update services independently, reducing the risk of system-wide issues.
They also improve fault isolation. If one service fails, it doesn't necessarily affect others. This can lead to more robust and reliable applications overall.
Java in Microservices
Java is a popular choice for building microservices. It offers many tools and frameworks that make creating small, independent services easier. Developers can use Java to build fast and reliable microservices.
Why Java for Microservices
Java shines in microservices development. It's stable, secure, and widely used. Java has a large community that provides support and resources. The language is also known for its "write once, run anywhere" ability. This makes it great for different platforms.
Java's ecosystem includes many libraries for microservices. These help with common tasks like logging and monitoring. The language also supports concurrent programming. This is key for handling multiple requests in microservices.
Companies like Netflix and Amazon use Java for their microservices. This shows its strength in large-scale systems. Java's long-term support means it's a safe choice for businesses.
Frameworks and Libraries
Spring Boot is a top framework for Java microservices. It makes creating standalone apps quick and easy. Spring Boot handles many setup tasks automatically. This lets developers focus on writing business logic.
Spring Cloud is another useful tool. It helps manage distributed systems. It offers features like service discovery and load balancing. These are crucial for microservices architectures.
Other helpful libraries include:
- Netflix Eureka for service registry
- Resilience4j for fault tolerance
- Feign for easy HTTP clients
These tools make building robust microservices simpler. They handle common challenges in distributed systems. This allows teams to create scalable and maintainable services.
Setting Up Java Microservices
Setting up Java microservices involves project initialization and configuration management. These steps lay the groundwork for building scalable and maintainable microservices.
Project Initialization with Spring Initializr
Spring Initializr makes it easy to start a new Java microservices project. Go to start.spring.io and pick Java as the language. Choose Spring Boot as the project type.
Select the needed dependencies like Spring Web and Spring Data JPA. Pick Maven or Gradle as the build tool. Maven is common, but Gradle offers more flexibility.
Click "Generate" to download a zip file with the project structure. Unzip it and open in your IDE. The main class will have a @SpringBootApplication annotation.
This setup includes a pom.xml file for Maven projects or build.gradle for Gradle. These files list project dependencies and build settings.
Configuration Management
Proper config management is key for Java microservices. The application.properties file in the src/main/resources folder holds important settings.
Set up database connections, server ports, and logging levels here. For example:
server.port=8080
spring.datasource.url=jdbc:mysql://localhost:3306/mydb
For more complex setups, use Spring Cloud Config Server. This central place manages configs for all microservices.
Create a Git repo to store config files. Set up a Config Server microservice to serve these files. Other services can then fetch their configs from this server.
This approach allows for easy updates across all microservices. It also supports different configs for various environments like dev, test, and production.
Service Discovery and Registration
Service discovery and registration are key parts of microservices. They help services find and talk to each other. This makes it easier to build and run complex systems.
Eureka and Service Registry
Eureka is a popular tool for service discovery in Java microservices. It acts as a service registry. Services sign up with Eureka when they start. They tell Eureka their name and network location.
Other services can then ask Eureka for this info. This lets them find and use the services they need. Eureka keeps its list up to date. It removes services that stop working.
Netflix made Eureka. It's now part of Spring Cloud. This makes it easy to use in Spring Boot apps.
Client-Side Discovery with Ribbon
Ribbon is another Netflix tool. It works with Eureka for client-side service discovery. Ribbon gets service info from Eureka. It then picks which instance to use.
This is called load balancing. It spreads work across many service instances. This helps systems handle more traffic and stay up if some parts fail.
Ribbon can use different ways to pick services. It can choose randomly or use round-robin. It can also check if services are healthy before using them.
Inter-Service Communication
Microservices need to talk to each other. They use different ways to share data and work together. Some methods are quick, while others can handle delays.
Synchronous vs Asynchronous
Synchronous communication is direct and fast. Services wait for replies before moving on. It's like a phone call. REST APIs often use this method.
Asynchronous communication doesn't need instant responses. Services send messages and keep working. It's like email. This works well for tasks that take time.
Each type has its place. Sync is good for quick data needs. Async helps with complex jobs that might fail.
REST APIs and Messaging
REST APIs are common in microservices. They use HTTP and are easy to understand. Services can request data or actions from each other.
REST works well for simple queries and updates. It's stateless, which means each request is separate.
Messaging systems help with async tasks. They use message queues to pass data between services. This is good for events and background jobs.
RabbitMQ and Apache Kafka are popular messaging tools. They can handle lots of messages and make sure they're delivered.
Service-to-Service Collaboration
Java microservices need ways to work together smoothly. Two key tools help them talk to each other and stay running even when things go wrong.
API Gateway
An API Gateway acts as a single entry point for client requests. It routes traffic to the right microservices and can handle tasks like security and rate limiting. This setup makes life easier for developers and users.
API Gateways can:
- Combine responses from multiple services
- Transform protocols between clients and services
- Offload common tasks like authentication
Popular Java API Gateway options include:
- Spring Cloud Gateway
- Netflix Zuul
These tools help manage the flow of data between services and outside users.
Load Balancing and Fault Tolerance
Load balancing spreads work across multiple servers. This helps services handle more traffic and stay up if one server fails. Fault tolerance keeps the system running even when parts break down.
Key concepts:
- Load balancers distribute requests evenly
- Circuit breakers stop calls to failing services
- Resilience4j helps build fault-tolerant systems
RabbitMQ can queue messages between services. This lets them keep working even if some parts are slow or down.
These tools make Java microservices more robust and able to handle problems.
Microservices Security
Securing microservices is crucial for protecting sensitive data and maintaining system integrity. Key focus areas include authentication, authorization, and secure communication between services.
Authentication and Authorization
Authentication verifies user identity, while authorization controls access to resources. For Java microservices, Spring Security offers robust solutions. It supports role-based access control, allowing fine-grained permissions for different users and services.
JSON Web Tokens (JWTs) are a popular choice for authentication in microservices. They contain user info and are passed between services, reducing the need for repeated logins. Spring Security can easily integrate JWT authentication.
Many systems use a central identity provider to manage user credentials across services. This approach simplifies user management and ensures consistent security policies.
Secure Communication
Microservices must communicate securely to protect data in transit. HTTPS is essential for encrypting traffic between services and clients. It uses TLS/SSL protocols to prevent eavesdropping and tampering.
API gateways act as a single entry point for external requests. They handle authentication, rate limiting, and can encrypt internal traffic. This adds an extra layer of security to the microservices architecture.
For internal service-to-service communication, mutual TLS (mTLS) is often used. It ensures both the client and server authenticate each other, preventing unauthorized access between services.
Deployment and Containerization
Deploying Java microservices involves packaging applications into containers and managing them with orchestration tools. This approach improves scalability and portability.
Docker and Kubernetes
Docker is a popular tool for containerizing Java microservices. It packages apps and their dependencies into portable units called containers. To use Docker:
- Create a Dockerfile with instructions for building the image
- Build the Docker image
- Run the container from the image
Kubernetes manages containerized applications across multiple hosts. It handles:
- Scaling
- Load balancing
- Self-healing
Kubernetes uses YAML files to define deployments, services, and other resources. It groups containers into pods and manages them as a single unit.
Continuous Deployment
Continuous deployment (CD) automates the process of releasing code changes to production. It helps teams deliver updates faster and more reliably.
Key steps in a CD pipeline for Java microservices:
- Build the application
- Run automated tests
- Create a Docker image
- Push the image to a registry
- Deploy to Kubernetes
Popular CD tools for Java microservices include Jenkins, GitLab CI, and CircleCI. These tools integrate with Docker and Kubernetes to streamline the deployment process.
CD best practices:
- Use feature flags to control new functionality
- Monitor deployments closely
- Have a rollback plan in case of issues
Monitoring and Maintenance
Keeping Java microservices running smoothly requires effective monitoring and maintenance. Two key approaches are distributed tracing and centralized logging.
Distributed Tracing with Zipkin
Zipkin helps track requests as they move through microservices. It shows how long each service takes to respond. This makes it easier to find slow parts of the system.
Zipkin uses unique IDs to follow requests. It records when a request starts and ends at each service. This data creates a map of the request's path.
Developers can see which services are causing delays. They can also spot errors and failed requests. Zipkin has a web interface to view and search traces.
Logging and the ELK Stack
The ELK Stack centrally manages logs from all microservices. ELK stands for Elasticsearch, Logstash, and Kibana.
Logstash collects logs from different sources and formats them into a standard style. Meanwhile, Elasticsearch stores and indexes these logs. Lastly, Kibana creates dashboards and graphs from the data.
This setup lets teams search logs across all services at once. They can set up alerts for errors or unusual patterns. Kibana's visuals help spot trends over time.
Using ELK makes it easier to debug issues spanning multiple services. It also helps teams understand how their system behaves as a whole.
Scaling Microservices
Scaling microservices is key for handling increased workloads and traffic. It allows systems to grow and adapt to changing needs.
Horizontal Scaling Strategies
Horizontal scaling adds more instances of a microservice to spread the load. This can be done by creating copies of the service on different servers or containers. Load balancers distribute requests across these instances.
Auto-scaling tools can add or remove instances based on demand. This keeps the system responsive during traffic spikes. It also saves resources when traffic is low.
Service discovery tools like Eureka help manage scaling. They keep track of available service instances. This lets other services find and use the right instances as the system grows.
Handling Distributed Transactions
Distributed transactions span multiple microservices. They need special care to maintain data consistency across services.
The Saga pattern is useful for managing these transactions. It breaks a big transaction into smaller steps. Each step can be undone if needed.
Two-phase commit is another option. It ensures all services agree before finalizing a transaction. But it can slow things down and create bottlenecks.
Event-driven architectures can help. They use message queues to keep services in sync. This approach is more flexible and can scale better than traditional transactions.
Best Practices and Tools
Java microservices benefit from proven patterns and powerful tools. These help create robust, scalable systems. They also speed up development and make maintenance easier.
Resilience Patterns
Circuit breakers stop cascading failures, cutting off faulty services to prevent system-wide issues. Meanwhile, retry mechanisms help handle temporary network glitches by automatically trying failed requests again.
Timeouts prevent long waits for unresponsive services by canceling requests after a set time. On the other hand, bulkheads isolate parts of the system to stop problems from spreading.
Lastly, rate limiting protects services from overload by capping the number of requests a service handles. Meanwhile, caching improves speed and reduces load on backend services by storing common data for quick access.
Microservices Development Tools
Spring Boot is a popular Java framework for microservices. It offers auto-configuration and embedded servers. This makes setup fast and easy.
Docker containers package microservices with their dependencies. This ensures consistent behavior across environments.
Kubernetes manages these containers at scale. It handles deployment, scaling, and load balancing.
Maven and Gradle are build tools that manage dependencies. They automate the build process.
Jenkins and GitLab CI enable continuous integration and delivery. These tools run tests and deploy code automatically.
Monitoring tools like Prometheus track system health. They collect metrics from microservices.
Logging frameworks such as SLF4J help track errors and debug issues. They provide insight into system behavior.