Optimizing Node Architecture for High Performance Web Applications
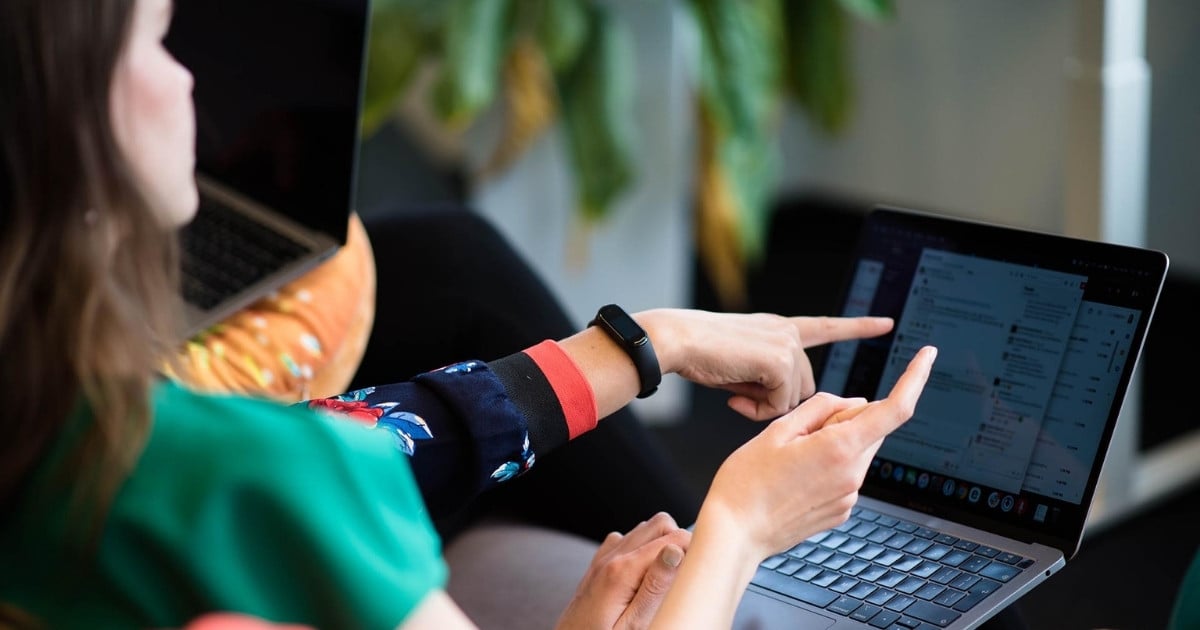
Key Takeaways
-
Node.js utilizes a single-threaded event loop architecture and a non-blocking I/O model, enabling efficient processing of multiple concurrent requests while minimizing server resource usage.
-
Key components such as the V8 engine, Libuv, and NPM enhance Node.js’s performance, allowing for high-speed execution, cross-platform support for asynchronous I/O, and easy management of dependencies.
-
Adhering to best practices, such as using linters, modularizing code, and implementing robust error handling, is essential for maintaining the quality and scalability of Node.js applications.
Understanding Node.js Architecture
Node.js operates on a single-threaded event loop architecture, which is pivotal for handling multiple concurrent requests with remarkable efficiency. Unlike traditional server-side platforms that create multiple threads to handle simultaneous client requests, Node.js leverages an event-driven, non-blocking I/O model to maintain high performance and scalability. This architecture allows Node.js to process a vast number of requests using minimal memory and server resources, making it an ideal choice for I/O-intensive applications such as chat apps and multimedia streaming services.
At the heart of Node.js is the V8 engine, an open-source JavaScript engine developed by Google. Built on Chrome’s V8 engine, Node.js optimizes JavaScript execution for high performance. The V8 engine compiles JavaScript directly into machine code, ensuring faster execution times and efficient memory usage. This powerful combination of the V8 engine and the event loop and js architecture is what makes Node.js a formidable platform for building high-performance web applications.
Another critical aspect of Node.js architecture is its non-blocking I/O model. Traditional server-side platforms often face challenges when dealing with multiple I/O operations simultaneously, leading to performance bottlenecks. Node.js, however, employs asynchronous operations that prevent blocking and enable the server to handle multiple tasks concurrently. This non-blocking approach minimizes lag and enhances the responsiveness of web applications, providing a seamless user experience.
Node.js’s architecture is not just about handling I/O operations efficiently; it also focuses on scalability. The ability to process requests asynchronously and manage multiple client connections simultaneously makes Node.js a preferred choice for developers looking to create fast and responsive web applications. With its robust js server architecture node, Node.js continues to set new standards for server-side development.
Single-Threaded Event Loop
The single-threaded event loop is the cornerstone of Node.js, enabling it to manage multiple client requests concurrently. This unique architecture allows Node.js to handle several operations simultaneously without creating multiple threads, which would consume additional resources. The event loop continuously checks for pending events and processes them, ensuring efficient handling of tasks and maintaining high performance.
Next, we delve deeper into the mechanics of the event loop, beginning with the Event Queue and then exploring the role of Callback Functions in managing asynchronous operations.
Event Queue
The Event Queue in Node.js plays a crucial role in managing incoming requests before they are processed by the event loop. When a client interacts with a Node.js server, their requests are added to the Event Queue, where they are stored in the order they are received. This queue ensures that all incoming client requests are efficiently managed and processed sequentially.
Once the event loop receives a request from the Event Queue, it determines whether the request requires external resources, such as accessing a database or reading a file. Simple requests that do not need external resources are processed immediately, while more complex tasks are delegated to the appropriate handler, ensuring that the server remains responsive.
Callback Functions
Callback functions are integral to Node.js’s asynchronous programming model. They are executed after an asynchronous operation is completed, allowing the main thread to continue processing other requests without waiting for the operation to finish. This approach prevents the event loop from being blocked and ensures that Node.js can handle multiple tasks simultaneously through the javascript callback mechanism.
Promises offer a more structured way to manage asynchronous operations, helping developers avoid the common problem of callback hell. Promises help developers write cleaner and more maintainable code, enhancing overall efficiency and readability.
Non-Blocking I/O Model
Node.js’s non-blocking I/O model is a game-changer for web application performance. Traditional server-side platforms often struggle with blocking I/O operations, where the server waits for a particular task to complete before moving on to the next one. In contrast, Node.js allows other tasks to continue executing while waiting for I/O operations to finish, thanks to its non-blocking approach.
This model relies heavily on asynchronous callbacks or promises to manage I/O operations in the background. By keeping the main thread free to handle other tasks, Node.js significantly reduces latency and improves the responsiveness of web applications. This makes it particularly well-suited for applications that require real-time updates and high concurrency, utilizing the javascript event based model and the js processing model.
Key Components of Node.js Architecture
The backbone of Node.js architecture comprises three key components: the V8 engine, Libuv, and the Node Package Manager (NPM). The V8 engine, developed by Google, is responsible for compiling JavaScript code, managing memory allocation, and performing garbage collection. Its ability to execute JavaScript code at high speed is one of the reasons behind Node.js’s exceptional performance.
Libuv provides a multi-platform support library designed for asynchronous I/O, enabling Node.js to access OS-level functionalities such as file system operations, network communication, and threading. This library abstracts the underlying operating system, allowing Node.js to run seamlessly across different platforms.
NPM, the Node Package Manager, is another critical component of Node.js architecture. It manages packages and dependencies in Node.js projects, making it easy for developers to include third-party libraries and modules in their applications. Together, these components enhance the performance and scalability of Node.js, making it a powerful platform for developing web applications.
Workflow of a Node.js Server
The workflow of a client browser Node.js web server begins when clients send requests to the server. These requests can range from simple data retrieval to more complex operations requiring external resources. The Event Loop sequentially manages these requests from the Event Queue, determining whether they need external resources or can be processed immediately.
For complex requests, a dedicated thread from the Thread Pool is allocated to handle multiple client requests. This ensures that the main event loop remains free to process other incoming requests, blocking client requests and maintaining the server’s responsiveness. After the task is completed, the response is sent back through the Event Loop to the respective client, ensuring efficient handling of client interactions.
To further improve throughput, Node.js can utilize clustering, which allows multiple concurrent client requests to handle requests simultaneously across CPU cores. This approach enhances the server’s ability to manage high traffic and ensures seamless scalability.
Design Patterns in Node.js
Design patterns play a crucial role in structuring Node.js applications for better maintainability and scalability. One popular architectural style is microservices, which structures an application as a collection of loosely coupled services. This approach allows individual services to be scaled independently, improving overall application performance.
Another important design pattern in Node.js is the singleton pattern, which ensures that a class has only one instance. This is particularly useful for managing shared resources like database connections, ensuring efficient resource utilization and reducing the risk of conflicts.
Best Practices for Node.js Development
Adhering to best practices in Node.js development is essential for maintaining code quality and ensuring the long-term success of your applications. Using linters and formatters helps detect bugs and enforce coding standards, resulting in cleaner and more maintainable code. Additionally, following style guides and writing comprehensive comments can significantly improve code readability and collaboration among team members.
Dividing your code into various modules promotes reusability and simplifies debugging and testing. Implementing a centralized error-handling component can streamline the process of managing and logging errors across different parts of your application. Unit testing is another critical practice, as it verifies the accuracy and correctness of individual code units.
To optimize performance, prefer asynchronous APIs and minimize unnecessary I/O operations. Middleware functions can also enhance the modularity and functionality of your Node.js applications, allowing you to implement various processing steps for incoming requests.
Utilizing Third-Party Libraries
Third-party libraries can significantly speed up development by providing pre-built functionalities that developers can leverage. NPM, the Node Package Manager, plays a crucial role in managing these packages and dependencies, making it easy to install and update third-party modules in your Node.js projects.
However, it is essential to strike a balance when using third-party libraries. Over-reliance on them can introduce numerous dependencies, potentially complicating maintenance and increasing the risk of security vulnerabilities. A balanced approach ensures that you can leverage the benefits of third-party libraries while minimizing potential risks.
Implementing Security in Node.js Applications
Security is a critical aspect of any web application, and Node.js provides several tools and practices to help developers secure their applications. The dotenv package, for example, allows developers to manage environment variables securely, storing sensitive information like API keys and database passwords in a .env file.
Using SSL/TLS protocols is another essential practice for enhancing the security of your Node.js applications. Additionally, utilizing the crypto API’s timingSafeEqual function can protect against timing attacks by ensuring constant-time comparisons.
Performance Optimization Techniques
Optimizing the performance of your Node.js applications involves several techniques and strategies. Gzip compression can significantly reduce load times by compressing files for quicker transfer. Implementing effective caching strategies can also lower latency by storing frequently accessed data in memory.
Application Performance Monitoring (APM) tools are invaluable for detecting performance issues and slow response times, allowing you to address bottlenecks proactively. Additionally, optimizing database queries and using indexes can enhance application performance.
Reducing the number of dependencies and employing load balancers to distribute incoming traffic across multiple servers are other effective strategies for improving performance.
Real-World Use Cases of Node.js
Node.js has proven its capabilities in various real-world applications. For instance, LinkedIn migrated its mobile backend to Node.js, achieving a 20-fold increase in performance. Similarly, PayPal unified its development environment with Node.js, resulting in a 35% reduction in average response time.
Other notable examples include Netflix, which reduced its application startup time by 70% after switching to Node.js for server-side rendering, and Uber, which implemented a real-time matching system using a js server to process millions of requests simultaneously.
These real-world use cases demonstrate the transformative impact of Node.js on application performance and scalability.
Summary
In summary, optimizing Node.js architecture involves understanding its core components, leveraging the single-threaded event loop, and implementing best practices for development and security. By adopting advanced design patterns and utilizing third-party libraries judiciously, developers can build high-performance web applications that are both scalable and resilient. The real-world examples of companies like LinkedIn, PayPal, and Netflix highlight the potential of Node.js to revolutionize web application development. Embrace these insights and unlock the full potential of Node.js for your next project.
Frequently Asked Questions
What is the single-threaded event loop in Node.js?
The single-threaded event loop in Node.js efficiently manages multiple client requests concurrently by continuously checking and processing pending events without the need for multiple threads. This architecture ensures high performance and effective task handling.
How does the non-blocking I/O model improve performance in Node.js applications?
The non-blocking I/O model in Node.js significantly improves performance by enabling the server to execute other tasks concurrently while awaiting I/O operations. This leads to reduced latency and heightened responsiveness, particularly beneficial for applications requiring real-time updates and managing high levels of concurrency.
What are the key components of Node.js architecture?
The key components of Node.js architecture are the V8 engine, which compiles JavaScript and manages memory, Libuv for asynchronous I/O operations, and NPM for package and dependency management. These components work together to enable efficient, non-blocking server-side applications.
Why is security important in Node.js applications, and how can it be implemented?
Security is vital in Node.js applications to safeguard sensitive data and preserve user trust. Implementation can be achieved through managing environment variables with the dotenv package, utilizing SSL/TLS protocols, and employing the crypto API's timingSafeEqual function to mitigate timing attacks.
What are some real-world examples of companies using Node.js?
LinkedIn, PayPal, Netflix, Uber, and Walmart are prominent companies using Node.js to enhance application performance and scalability, with LinkedIn experiencing a notable 20-fold performance increase after adopting it for their mobile backend. This showcases the effectiveness of Node.js in addressing high-demand processing needs.