Essential Node.js Design Patterns for Modern Developers
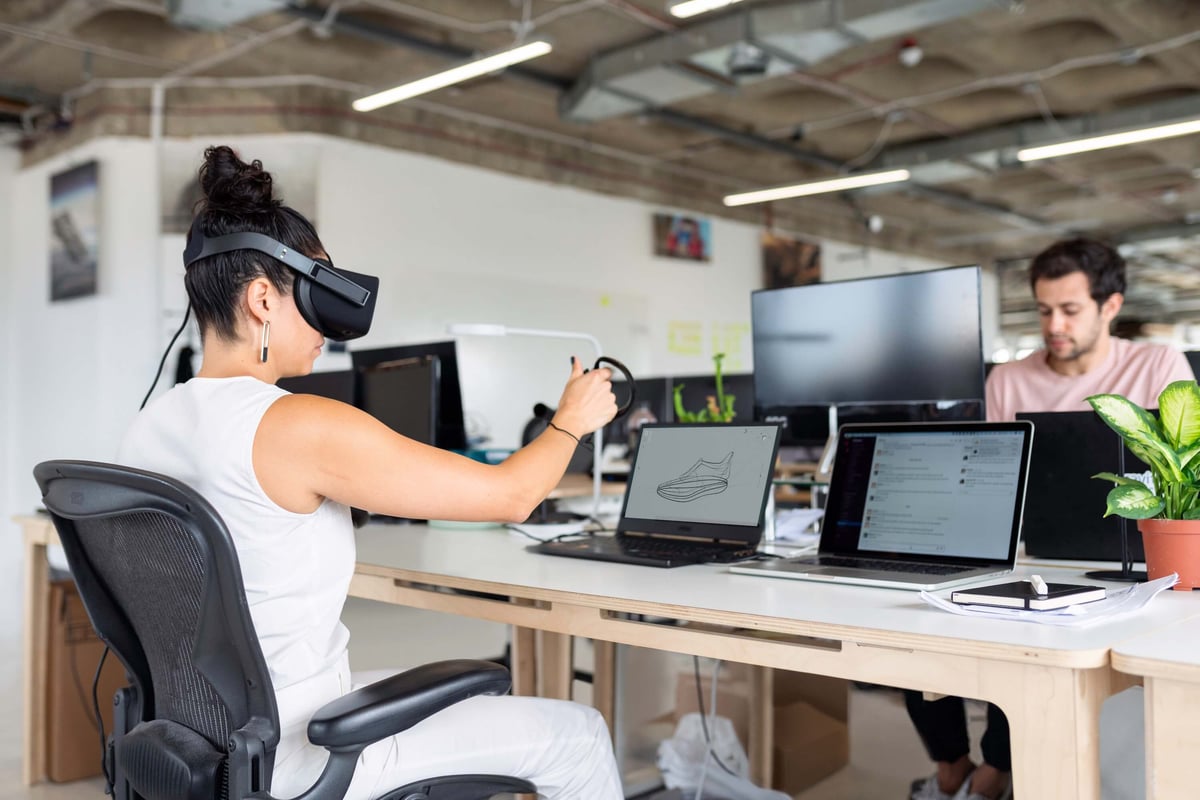
Key Takeaways
-
Design patterns in Node.js enhance code efficiency, scalability, and maintainability by providing reusable solutions for common issues.
-
Key design patterns like Singleton, Factory, and Observer facilitate better resource management, object creation, and event handling in applications.
-
Utilizing patterns such as Middleware and Streams helps promote modular architecture, ensuring smoother asynchronous operations and cleaner code organization.
Understanding Design Patterns in Node.js
Design patterns are reusable solutions to common software design problems. They provide a proven template for solving recurring issues, making your code more scalable, flexible, and maintainable. In Node.js, implementing these js design patterns can significantly improve your application’s architecture and overall quality.
One of the primary benefits of using design patterns in Node.js is the enhancement of coding efficiency. Design patterns accelerate development and encourage code reuse by providing optimal solutions to frequent problems. This approach speeds up the coding process and ensures that solutions are tried and tested, reducing the likelihood of bugs and errors.
Design patterns contribute to modular architecture, promoting better separation of concerns. Such modularity simplifies the codebase, making it easier to manage, understand, and maintain. Design patterns also facilitate better team communication by providing a common language for discussing solutions, invaluable in collaborative environments.
Understanding and implementing design patterns significantly enhances the scalability and maintainability of Node.js applications. They reduce code complexity, making it easier to introduce new features or modify existing ones without causing disruptions. Leveraging design patterns enables the building of robust applications that stand the test of time.
Singleton Pattern
The Singleton pattern is a fundamental design pattern that ensures only one instance of a class exists throughout the application. It provides a global access point for that instance, making it ideal for managing shared resources like database connections.
There are various methods to implement the Singleton pattern in Node.js. A common approach is creating a class with a static method that returns the existing instance or creates a new one if none exists. Alternatively, Immediately Invoked Function Expressions (IIFEs) or ES6 modules with a locally scoped variable can ensure only one instance is created and reused.
A typical use case for the Singleton pattern is managing database connections. The Singleton pattern reduces overhead and prevents conflicts and inconsistencies by ensuring only one connection is created and reused. This pattern is also useful in scenarios where a centralized resource or state must be consistently maintained across the application.
Factory Pattern
The Factory Pattern is another essential design pattern that serves as an abstraction for creating objects without specifying their exact classes. It provides an interface for object creation, ensuring a standard method for producing objects. This creational design pattern is particularly useful when the exact type of the object to be created isn’t known until runtime, making it a prime example of the factory design pattern.
A key benefit of the Factory Pattern is its ability to decouple object construction from the objects themselves. This promotes flexibility and maintainability, as changes to object creation logic are centralized within the factory, minimizing the impact on the rest of the code. The Factory Pattern also allows subclasses to alter the type of objects being produced, enhancing modularity.
In practice, the Factory Pattern can be used to define an interface or abstract class for creating objects, which subclasses then implement. This encapsulation of object creation logic makes the code easier to read and maintain, and it enhances testability by promoting loose coupling. The Factory Pattern is a powerful tool for managing interdependent or related classes in complex applications.
Builder Pattern
The Builder pattern is designed to separate the construction of complex objects from their representation, allowing developers to create objects step-by-step. This builder design pattern is particularly useful when dealing with complex objects that require detailed configuration.
With the Builder pattern, developers can set properties incrementally, avoiding the need to pass undefined or null values for omitted attributes during complex object instantiation. This approach enhances code readability and maintainability, making it easier to understand and modify object creation processes, especially when dealing with a new instance.
While the Builder pattern may be overkill for simple object creation, it is invaluable for more intricate scenarios.
Prototype Pattern
The Prototype pattern leverages prototypal inheritance to share properties among multiple objects of the same type. Instead of creating instances of a class, this pattern involves cloning existing objects, allowing new objects to inherit properties from the prototype design pattern.
Developers can implement the Prototype pattern in Node.js using the Object.create method to create a new object with a specified prototype. The clone method can also be employed to copy an existing object’s properties, thereby creating new objects efficiently.
A significant benefit of the Prototype pattern is its ability to reduce memory usage and improve performance by referencing shared methods within objects. It also avoids redundant code, making applications more efficient and easier to maintain. The Prototype pattern is particularly useful for caching data and creating new objects based on a prototype.
Observer Pattern
The Observer pattern is a powerful design pattern that enables one-to-many dependencies, where multiple observers react to changes in a single observable object. This pattern is closely related to event handling and is particularly useful for handling asynchronous events in Node.js.
Real-time updates and triggering events, such as push notifications, are common use cases for the Observer pattern. The EventEmitter class in Node.js facilitates the implementation of this pattern by allowing multiple functions to act as listeners for specific events. This capability makes the Observer pattern essential for creating responsive and real-time applications.
Dependency Injection Pattern
Dependency injection is a design pattern used to decouple application components, enhancing modularity and testability. Injecting dependencies rather than creating them internally promotes loose coupling and allows for easier testing and maintenance.
Various techniques exist for implementing dependency injection in Node.js. These include constructor injection, setter injection, and interface injection. Each method provides a way to inject dependencies, ensuring that components remain independent and easily testable.
A significant benefit of dependency injection is the ability to switch implementations without modifying the dependent class. Such flexibility is crucial for developing enterprise-grade distributed applications, where different implementations may be required for different environments. It also simplifies unit testing by allowing classes to be tested without real instances, further enhancing testability.
Middleware Pattern
The Middleware pattern is a cornerstone of Node.js development, particularly in frameworks like Express.js. Middleware functions perform tasks between API requests and responses, enhancing modularity and scalability. These functions can be utilized for various purposes, including authentication, logging, and error handling.
In Express.js, middleware can modify the request object and create a layered processing structure, enabling flexible and efficient data handling. Error-handling middleware is unique in that it takes four arguments, allowing it to handle errors effectively in the request-response cycle.
Promoting loose coupling, the Middleware pattern allows multiple handlers to manage requests without direct knowledge of each other, contributing to cleaner code and separation of concerns.
Asynchronous Control Flow Patterns
Managing asynchronous operations in Node.js can be challenging, especially with the risk of ‘callback hell,’ where excessive nesting makes the code difficult to read and maintain. Asynchronous control flow patterns provide strategies to handle these challenges effectively.
Organizing functions into an initiator, middleware, and terminator structure helps manage complex asynchronous operations. This approach allows for better state management and flexibility in handling asynchronous tasks. Asynchronous operations can be executed in series, in parallel, or with limited parallelism, depending on the specific requirements of the task.
In Node.js, the promise pattern is widely used for handling asynchronous operations sequentially. Promises help avoid callback hell and offer a more structured way to manage asynchronous tasks. Such patterns are crucial for building efficient and maintainable Node.js applications.
Module Pattern
The Module pattern is designed to separate and encapsulate code into different modules, promoting better organization and maintainability. Using closures, this pattern creates components that encapsulate both private and public elements, minimizing the risk of variable and function name collisions.
Modules can reveal only necessary functionalities while safeguarding internal details, enhancing reuse and abstraction. Reducing reliance on global variables, this approach prevents conflicts and contributes to cleaner code. The Module pattern ensures data encapsulation, protecting it from external access and making the codebase more secure and maintainable.
Immediately Invoked Function Expressions (IIFE)
Immediately Invoked Function Expressions (IIFEs) are a clever way to encapsulate code and avoid global scope pollution in JavaScript. As their name suggests, IIFEs are functions that are executed immediately after they are defined. The structure of an IIFE includes an anonymous function declaration inside parentheses, followed by calling parentheses which invoke the function immediately.
IIFEs encapsulate variables and functions within a local scope, preventing conflicts with other code and maintaining a cleaner global namespace. This makes IIFEs essential for enhancing code readability and maintainability, especially in larger codebases where avoiding variable collisions is crucial.
Proxy Pattern
A Proxy pattern serves as a substitute or placeholder. This pattern controls access to another object. It is particularly useful for adding functionalities such as lazy loading, access control, and logging/debugging. Acting as an intermediary, the proxy ensures that only authorized interactions with the target object are permitted, enhancing security and control.
The Proxy pattern in Node.js can be implemented using the Proxy object introduced in ECMAScript 6. This object allows developers to define custom behavior for fundamental operations such as property lookup, assignment, and function invocation. Leveraging the Proxy pattern allows managing access control, implementing caching mechanisms, and tracking object interactions for debugging purposes.
Developers should be mindful of the trade-offs involved in using proxies. Overuse or unnecessary complexity can undermine maintainability despite their significant advantages. Combining the Proxy pattern with other design patterns like Observer and Decorator can lead to more effective and robust solutions.
Chain of Responsibility Pattern
The Chain of Responsibility pattern decouples request senders from receivers by passing requests through a series of handlers. Each handler decides whether to process the request or pass it to the next handler in the chain. It is particularly useful in scenarios requiring dynamic assignment of request handling responsibilities.
This pattern simplifies complex logic by allowing request processing to flow through a chain of handlers, each responsible for specific aspects of the request. Key components of the Chain of Responsibility pattern include a handler interface, concrete handlers, and a client that initiates requests.
Streams
Streams in Node.js efficiently handle large amounts of data in chunks, making them ideal for processing data that might not fit into memory all at once. They can be categorized into four essential types: Writable, Readable, Duplex, and Transform.
Readable streams emit ‘data’ events when they have data chunks available for consumption, while writable streams allow data to be written to them and can emit events like ‘finish’ and ‘error’. Duplex streams serve as both readable and writable, enabling two-way data transfer, and transform streams can modify data as it is being read or written.
Node.js streams operate in two modes: flowing and paused. In flowing mode, data is read from the stream as soon as it is available, while in paused mode, data is read manually. The stream.pipelines API simplifies managing stream consumption and allows for better error handling.
A crucial aspect of working with streams is backpressure management. It involves controlling the data flow based on the processing rate to avoid overwhelming memory. The pipeline model, which includes stages of read streams, transform streams, and write streams, helps manage backpressure effectively, ensuring efficient data handling.
Summary
In summary, design patterns play a vital role in modern Node.js development. From the Singleton and Factory patterns to more advanced concepts like Dependency Injection and the Proxy pattern, each pattern offers unique benefits that can enhance your application’s scalability, maintainability, and efficiency.
By implementing these patterns, you can create robust, modular, and well-structured applications that stand the test of time. Embrace these design patterns in your development workflow, and you’ll find yourself equipped with powerful tools that not only solve common problems but also elevate your coding standards.
Frequently Asked Questions
What is the primary benefit of using the Singleton pattern?
The Singleton pattern is great because it guarantees only one instance of a class exists, giving you a single point of access to manage shared resources efficiently. This can simplify your code and help prevent resource conflicts.
How does the Factory pattern enhance maintainability?
The Factory pattern boosts maintainability by separating object creation from their use, making it easier to update and modify code without impacting other areas. This way, you can tweak your creations as needed while keeping everything else intact.
What are the key components of the Builder pattern?
The Builder pattern mainly consists of a director that manages the construction process, a builder interface or abstract class, and concrete builder classes that define how to create the various parts of the object. This separation helps you build complex objects step by step while keeping your code flexible and scalable.
How does the Observer pattern handle asynchronous events?
The Observer pattern efficiently manages asynchronous events by enabling multiple observers to respond to updates from a single observable object, making it perfect for real-time scenarios like those in Node.js. This way, changes can be communicated seamlessly without blocking the main thread.
Why is the Module pattern important in Node.js?
The Module pattern is crucial in Node.js as it helps keep your code organized and maintainable by encapsulating functionality in modules, minimizing global variable use, and improving readability and security. By using this pattern, you’re setting yourself up for cleaner and more manageable code.