Building a Robust Node.JS WebSocket Server: A Comprehensive Guide
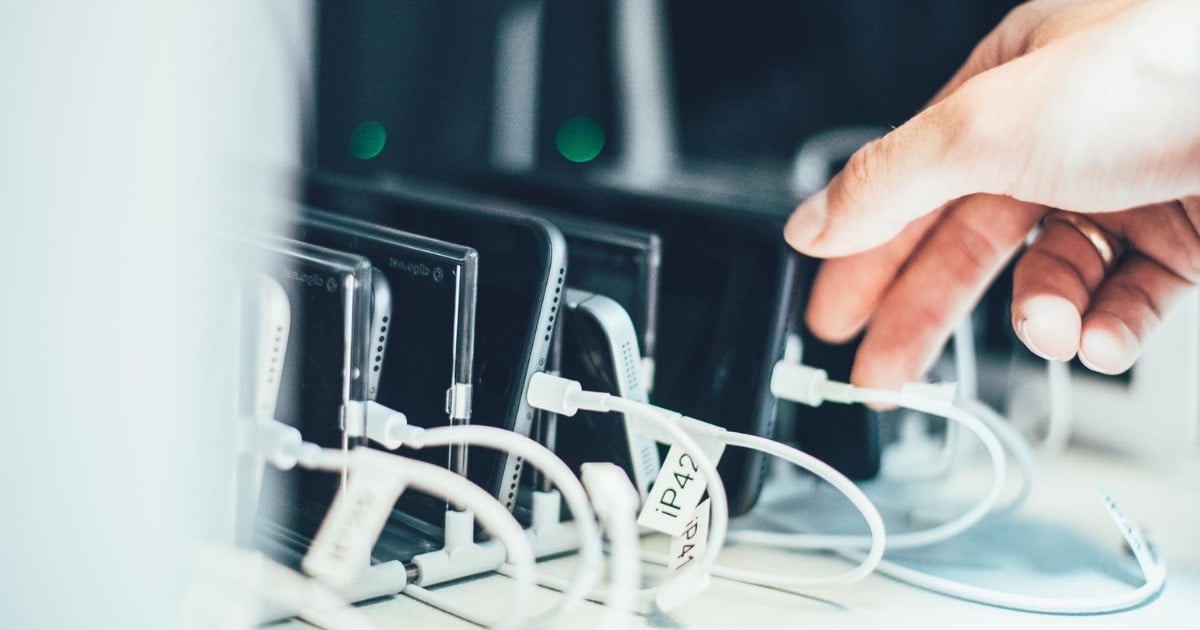
Key Takeaways
-
WebSockets enable bidirectional communication for real-time data exchange, enhancing user experience in applications like chat and live data feeds.
-
Setting up a WebSocket server in Node.js involves installing necessary libraries like ‘ws’, creating a server file, and handling client connections effectively.
-
To ensure performance and scalability, consider using horizontal scaling techniques and solutions like Redis for state management, alongside secure deployment practices.
Understanding WebSockets in Node.js
WebSockets revolutionize web communication by enabling full-duplex communication, allowing data to flow simultaneously in both directions, creating dynamic exchanges between client, server, and WebSocket object.
WebSocket servers built on Node.js leverage its non-blocking, event-driven architecture, making it ideal for real-time applications. Enhanced built-in support for WebSockets in Node.js simplifies development, providing a fast and scalable environment for handling connections.
What are WebSockets?
WebSockets upgrade traditional HTTP by enabling persistent connections for real-time data exchange. Unlike HTTP, which requires client requests for data, WebSockets establish a full-duplex connection on top of TCP, allowing continuous data exchange without reopening connections.
This bidirectional communication between client and server ensures that data flows seamlessly, providing real-time updates and enhanced user experiences.
Benefits of Using WebSockets
WebSockets offer vast benefits, especially for low-latency communication applications. They facilitate fast, bidirectional communication, making them ideal for real-time applications like chat and live data feeds. By maintaining a single connection for multiple exchanges, WebSockets reduce overhead and support efficient data transmission by eliminating repeated connection setups.
This makes WebSockets perfect for applications requiring real-time data exchange, such as games, stock tickers, and notifications.
Setting Up Your Development Environment
To start building a WebSocket server, download and install Node.js, which includes npm by default. Initialize a new Node.js project by running npm init
in your terminal and following the prompts to set the package details.
Next, install the Express framework and the ws library for WebSockets by running npm install express ws
in your project directory. Ensure that your project setup is correct by checking the package.json file and confirming all necessary dependencies are listed.
Installing Node.js and npm
To create a WebSocket server in Node.js, install Express.js and the ws library. Visit the official Node.js website, download the installation package, run the installer, and follow the on-screen instructions.
npm, the Node Package Manager, is automatically installed with Node.js. Verify the installation by typing node -v
and npm -v
in your command line.
Initializing a Node.js Project
Start a new Node.js project with the ‘npm init’ command to set up package metadata. Designate the main entry point for your application, commonly as ‘server.js’.
After initializing, add the WebSocket library as a dependency by running npm install –save ws
. This includes the ‘ws’ library in your project, essential for WebSocket functionality. Node.js’s npm integration ensures smooth dependency handling.
Building a Basic WebSocket Server
Creating a basic WebSocket server involves developing both the server and client components, crucial for applications like chat systems where maintaining client connections is essential.
WebSocket servers maintain client connections by sending periodic ping messages and expecting pong responses, keeping the connection active and ensuring smooth real-time communication.
Let’s dive into the steps to create your WebSocket server.
Creating the WebSocket Server
Begin creating a WebSocket server by installing the ‘ws’ package using npm. In your project directory, run ‘npm install ws’. Next, create an ‘index.js’ file to act as the server-side JavaScript file.
Inside this file, initiate an instance of WebSocketServer with the desired port, commonly 7071. This sets up your WebSocket server to listen for incoming connections, handling WebSocket functionality and maintaining the real-time communication channel between client and server.
Handling WebSocket Connections
When a new client connects, the ‘connection’ event triggers, allowing you to manage the interaction. Use a Map to maintain user state, tracking connected WebSockets and their metadata, enabling you to handle client-specific actions and maintain persistent connections.
Handle disconnections by listening to the ‘close’ event emitted when a client disconnects, ensuring resources are properly cleaned up and the server continues running smoothly.
Implementing WebSocket Client-Side Code
Client-side code is crucial for interacting with your WebSocket server. Set up HTML and JavaScript files to establish the WebSocket connection and handle message communication. Implement a ‘ping/pong’ mechanism to ensure the client remains connected by verifying the server’s response.
Let’s explore the steps to set up your client-side environment and enable real-time communication with the server.
Setting Up HTML and JavaScript Files
Create a simple html page structure to set up a WebSocket client. Create an HTML file and include a script tag that points to a js file named ‘script.js’. Here is a code example.
In ‘script.js’, use the WebSocket constructor to set up a connection with a valid server URI. Implement the connectToServer()
function to initiate the connection. This setup allows the client to establish a WebSocket connection and prepare for real-time data exchange.
Sending and Receiving Messages
To send a message to the server, call the send method on the WebSocket instance. The client can listen for messages from the server by defining an onmessage event handler, which processes incoming messages and updates the client accordingly.
Messages will appear in both user’s browser almost simultaneously, ensuring seamless real-time communication between client and server.
Enhancing Your WebSocket Server
Enhance your WebSocket server by implementing advanced features to increase robustness and performance. Add message authentication, connection monitoring, and load balancing to improve management, enhance security, and ensure a better user experience.
Let’s look at some specific techniques to enhance your WebSocket server.
Broadcasting Messages to All Clients
Maintain an array of connected clients to achieve broadcasting in Node.js. This allows the server to track all active WebSocket connections. When a client sends a message, the server loops through connected clients and pushes the message to each one.
Broadcast messages from one client to all others by sending the same message to multiple connected clients through the WebSocket server, ensuring simultaneous updates.
Error Handling and Reconnection Strategies
Proper error handling in WebSocket code is crucial to prevent server crashes when connections fail. This often involves catching exceptions and implementing retries to reconnect after disconnections, commonly using a delay with exponential backoff.
Using a library like ReconnectingWebSocket can simplify managing reconnections without extensive custom logic, ensuring persistent connections and a robust server.
Scaling WebSocket Connections
Scaling WebSocket connections requires careful architecture planning to efficiently manage persistent connections. A Node.js server can handle tens of thousands of WebSocket connections, but deployment considerations must ensure high availability and low latency.
Vertical scaling increases server capacity but has a performance ceiling and can be expensive. Horizontal scaling shares the processing load across multiple servers, improving reliability and eliminating single points of failure.
Let’s explore these scaling strategies and how to implement them.
Vertical vs. Horizontal Scaling
Horizontal scaling distributes the processing load across multiple servers, improving reliability and eliminating single points of failure. This method involves adding more servers to handle the load and ensuring no single server becomes a bottleneck.
Vertical scaling, while simpler to implement, involves upgrading the existing server’s hardware to handle more connections, which can be costly and has scaling limits. Choose the right scaling method based on your application’s needs and infrastructure.
Using Redis for State Management
Redis is popular for maintaining shared state among multiple WebSocket server instances, facilitating communication. Use Redis to share session data, user states, and other critical information across server instances, ensuring consistency and allowing horizontal scaling while maintaining real-time data synchronization.
Implementing Redis in your WebSocket server architecture significantly enhances scalability and reliability.
Deploying Your WebSocket Server
Deploying your WebSocket server involves choosing the right hosting provider and setting up HTTPS for secure connections. Several providers offer features tailored to various needs, from user-friendly interfaces to automated scaling and SSL management.
Using a hosted real-time communication solution like PubNub or Ably saves time and resources by removing the need to build and maintain your own infrastructure. Ensuring high availability and low latency is crucial for successful deployment.
Choosing a Hosting Provider
Choosing the right hosting provider is critical for performance and scalability. RunCloud offers a user-friendly interface for managing Node.js applications on VPS, allowing flexibility across various cloud providers. Render simplifies deployment by automating SSL management and scaling, suitable for small to medium-sized applications.
Fly.io utilizes edge deployment to ensure Node.js applications run closer to users, enhancing performance and reducing latency. Other providers like Cloudflare, Railway, Heroku, and Vercel offer features to optimize your WebSocket server deployment.
Setting Up HTTPS
Implementing HTTPS for WebSocket connections is crucial for maintaining privacy and security during data transmission. HTTPS ensures the data exchanged between client and server is encrypted, protecting sensitive information from potential threats.
When setting up HTTPS for a WebSocket server, use ‘wss://‘ for secure connections, as browsers require secure WebSocket connections on HTTPS pages. Configure your web server to support HTTPS and ensure all WebSocket connections are established using the ‘wss://‘ URL scheme.
Common Use Cases for WebSockets
WebSockets are used in various applications requiring real-time updates and low latency, making them 5–7 times faster than HTTP and ideal for rapid data exchange scenarios. Applications like real-time chat, live data feeds, and notifications benefit greatly from their persistent connection and instant communication capabilities.
Let’s explore some common use cases to understand how WebSockets can enhance user experiences.
Real-Time Chat Applications
WebSockets enable seamless real-time messaging between users, allowing instant message exchange. This functionality is crucial for chat applications where instant communication is the core feature. WebSockets support features like typing indicators and read receipts, enhancing the interactive experience.
In a chat application, multiple users can see the same messages simultaneously, creating a dynamic and engaging communication environment.
Live Data Feeds and Notifications
WebSockets provide a persistent connection that allows real-time updates between the server and client. This capability is essential for applications that need to push live data to users instantly, such as stock tickers and sports scores. WebSockets eliminate the need for polling, reducing latency and enhancing the user experience.
Real-time notifications, another common use case, keep users engaged by providing instant updates about important events or changes.
Summary
WebSockets revolutionize real-time web communication by enabling full-duplex connections that facilitate instant data exchange. Node.js, with its non-blocking architecture, is an excellent platform for building scalable WebSocket servers. By setting up the development environment, creating and enhancing the WebSocket server, implementing client-side code, and deploying the server, you can create robust web applications that provide real-time interactions. With use cases ranging from chat applications to live data feeds, WebSockets offer immense potential for enhancing user experiences. Embrace the power of WebSockets and transform your web applications into dynamic, real-time platforms.
Frequently Asked Questions
What are WebSockets?
WebSockets provide a persistent, full-duplex communication channel that enhances real-time data exchange between clients and servers, effectively improving the performance of web applications. This technology is a significant upgrade over traditional HTTP connections.
How do you set up a WebSocket server in Node.js?
To set up a WebSocket server in Node.js, install Node.js and npm, create a new project using 'npm init', and utilize the 'ws' library for WebSocket functionality. This approach allows for real-time communication in your application.
What are the benefits of using WebSockets?
WebSockets enable low-latency, bidirectional communication, which significantly reduces overhead and supports efficient data transmission. This feature makes them particularly suitable for real-time applications such as chat and live data feeds.
How do you handle WebSocket connections and errors?
To effectively handle WebSocket connections, utilize the 'connection' and 'close' events for managing connections, while implementing error handling strategies such as retries and exponential backoff to ensure stability. This approach not only maintains persistent connections but also enhances overall reliability in communication.
What are some common use cases for WebSockets?
WebSockets are commonly used for real-time chat applications, live data feeds such as stock tickers, and real-time notifications, leveraging their low latency for instant data exchange. These use cases highlight the effectiveness of WebSockets in enhancing user experiences through immediate communication.