Essential Python Debugging Tools You Need to Know
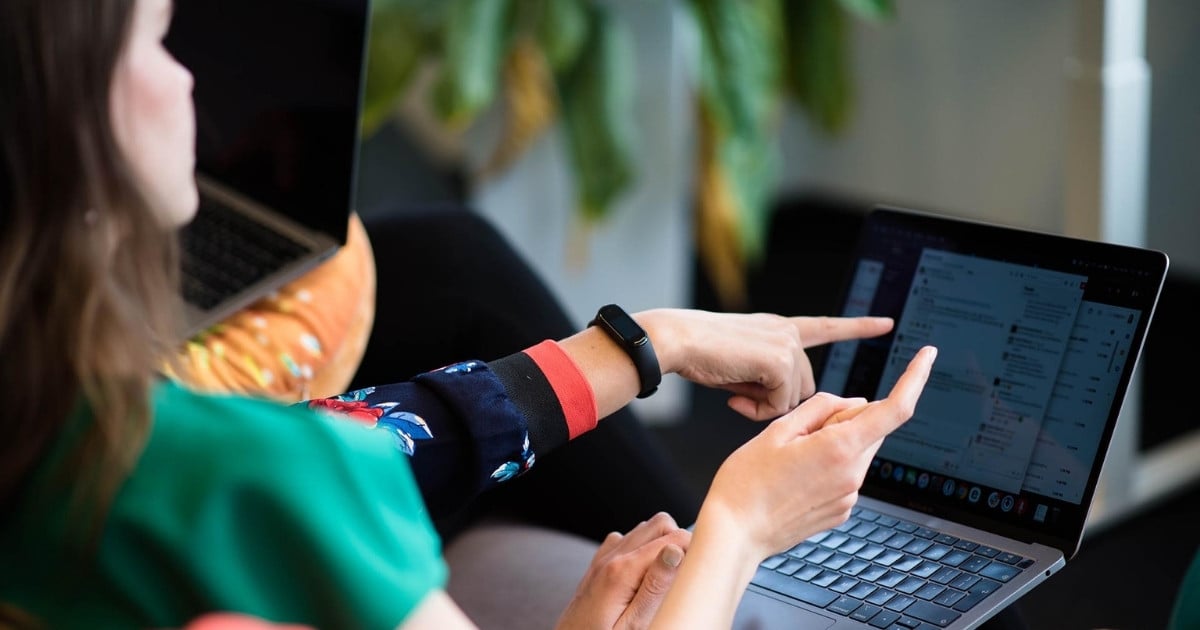
Key Takeaways
-
Effective debugging is essential for identifying and resolving errors in Python code, leading to improved code quality and performance.
-
Popular debugging tools include PDB, PyCharm Debugger, and Visual Studio Code Python extension, each offering unique features suited for different development needs.
-
Advanced techniques like remote debugging and post-mortem debugging enhance troubleshooting capabilities, allowing developers to address complex issues in various environments.
Understanding Python Debugging
Debugging involves identifying and fixing errors within Python code. It’s a critical skill for every developer, integral to the development cycle of any project, regardless of its complexity. Effective debugging allows developers to swiftly identify issues in their code, ensuring that programs behave as expected and output the correct results. This process is not just about finding bugs but also about understanding the program’s execution flow, which can lead to more informed decisions for code improvements.
Moreover, debugging can uncover performance issues by identifying inefficient code that may slow down execution. It also helps in removing obsolete code, including duplicate functions or unnecessary variables, which contributes to cleaner and more maintainable codebases.
Integrating effective debugging practices enhances the quality and reliability of Python programs, making debugging indispensable in Python development.
Popular Python Debuggers
Python debuggers are essential tools that help developers identify, isolate, and fix issues within their code, ultimately improving code quality and efficiency. From built-in command-line tools to feature-rich integrated development environments (IDEs), there are numerous options available for debugging Python code.
Here are some of the best python debugging tools catering to various preferences and development environments.
PDB - The Built-in Debugger
PDB is Python’s built-in command-line debugger, included in the standard library. It’s best suited for developers who are comfortable with command-line tools and prefer a straightforward approach to debugging. Start using PDB in a Python script by importing the pdb module and using the pdb.set_trace() statement to set breakpoints. This allows you to pause program execution and inspect variable values, providing a clear view of the program’s state at specific points.
While PDB might seem basic compared to more feature-rich debuggers, it offers powerful capabilities for those who prefer or need a command-line interface. Its simplicity and integration with the Python standard library make it an invaluable tool for quick and effective debugging.
PyCharm Debugger
PyCharm, a feature-rich IDE specifically designed for Python development, offers advanced debugging features that cater to both beginners and experienced developers. Its intuitive interface and comprehensive set of functionalities make it an excellent choice for debugging Python code. PyCharm’s debugger includes step-by-step execution, variable inspection, breakpoints, and remote debugging capabilities, significantly enhancing the developer’s ability to troubleshoot and refine code effectively.
These features are complemented by PyCharm’s intelligent code completion, syntax highlighting, and refactoring tools, which contribute to a seamless and efficient debugging process. Whether you’re debugging simple scripts or complex applications, PyCharm provides the tools you need to identify and fix issues quickly.
Visual Studio Code Python Extension
The Visual Studio Code Python extension is a versatile tool for Python development, integrating with various tools to cater to both novice and experienced developers. For debugging, the Python Debugger extension must be installed in Visual Studio Code, enabling features such as real-time variable inspection, step-by-step debugging, and breakpoints. Additionally, it supports specific debugging modes like ‘debug-test’ or ‘debug-in-terminal’, which can be configured in the launch.json file.
Breakpoints in Visual Studio Code can be set with various conditions, including expressions and hit counts, offering a flexible debugging environment. This, combined with the ability to handle subprocesses and other advanced debugging capabilities, makes Visual Studio Code a powerful tool for Python development.
Advanced Debugging Techniques
For more complex development environments, advanced debugging techniques are essential. These techniques include remote debugging and post-mortem debugging, which provide additional capabilities for troubleshooting and optimizing Python code.
Let’s delve into these advanced methods and see how they can enhance your debugging process.
Remote Debugging
Remote debugging is the process of debugging code running on a separate system or server, which is particularly useful when applications are deployed on remote servers, in the cloud, or on different devices. Tools like PyCharm and Visual Studio Code support remote debugging, allowing developers to step through a program locally while it runs on a remote computer. This involves setting remote breakpoints, stepping through code, and inspecting variables, which can be done securely using methods such as SSH tunnels to ensure data integrity.
Remote debugging eliminates the need to install the full development environment on the remote system, streamlining the debugging process and making it easier to troubleshoot issues in production environments. Remote debugging helps developers identify and fix bugs in distributed applications more effectively.
Post-Mortem Debugging
Post-mortem debugging enables the examination of a program’s state after an exception has occurred. This technique is invaluable for understanding what went wrong without having to reproduce the error. Analyzing the program’s state at the point of failure provides insights into the root cause, allowing developers to devise appropriate fixes.
Debugging Tools for Specific Use Cases
Certain debugging tools are tailored for specific scenarios, such as web applications and memory profiling. These specialized tools offer features that address the unique challenges of their respective use cases, making them indispensable for developers working in these areas.
Debugging Web Applications
For web applications, tools like Flask-DebugToolbar and Django Debug Toolbar provide essential debugging support. Flask-DebugToolbar, for example, enhances the debugging experience for Flask web applications by providing a detailed view of request variables, SQL queries, and template context.
Similarly, Django Debug Toolbar offers a suite of panels to inspect various aspects of Django applications, from template rendering to database queries, helping developers identify and resolve issues efficiently. These tools integrate seamlessly with their respective frameworks, offering real-time insights into the application’s behavior and making it easier to diagnose and fix bugs in web applications.
Memory Profiling
Understanding memory usage is crucial for optimizing code, especially in resource-constrained environments. The memory_profiler module is a powerful tool for profiling memory consumption in Python programs. It analyzes memory usage line-by-line during code execution, helping developers identify specific areas that contribute significantly to memory usage and optimize them accordingly.
Enhancing Debugging Efficiency
Good debugging habits save time and enhance the quality and reliability of code. By choosing the right debugging tools and practices, developers can significantly improve their debugging process and systematically identify and fix errors.
Let’s explore some strategies for enhancing debugging efficiency.
Using Print Statements Effectively
Strategically placed print statements can help isolate issues by revealing the flow of execution and confirming variable states. Incorporating formatted strings enhances clarity when displaying variable values, making it easier to track code behavior. This approach provides real-time checks on code behavior and can significantly improve the debugging process.
However, it’s important to remove or comment out print statements once the debugging process is complete to avoid cluttering the code and affecting performance.
Leveraging Logging
Using logging instead of print statements provides a structured way to track program activities without cluttering the code. Logging enables detailed records of application activity, improving error tracking and debugging by offering insights into code execution and variable states. This method is particularly useful for long-running applications and production environments, where continuous monitoring is essential.
Implementing a robust logging strategy helps developers effectively debug their code and maintain a clear record of program activities for future reference.
IDE Features for Debugging
Popular Python IDEs offer integrated debugging features that enhance developers’ workflow and improve code quality. These features include visual breakpoints, variable inspection, step-by-step execution, and more, all designed to streamline the debugging process.
Breakpoints and Conditional Breakpoints
Breakpoints are markers set in the code to pause execution for debugging purposes, allowing developers to inspect the state of the application at specific points. In Visual Studio Code, breakpoints can be easily toggled by clicking in the editor margin or using a keyboard shortcut, and conditional breakpoints can be set to activate only when a specific expression evaluates to true.
This feature enables more targeted debugging and helps developers quickly identify and fix issues.
Variable Inspection and Watch Expressions
Variable inspection tools, such as the VARIABLES panel in Visual Studio Code, allow developers to view and modify variable states during debugging sessions. Watch expressions enable continuous monitoring of variable changes as the program executes, providing real-time insights into the program’s behavior. Developers can also inspect variables to gain a deeper understanding of their applications.
These features are invaluable for understanding how data changes during execution and identifying unexpected behaviors.
Command Line Debugging
For developers who prefer command-line tools, Python offers robust command-line debugging capabilities. The Python debugger can be launched directly from the command line using the ‘-m pdb’ option, allowing developers to run a Python script and start the debugger automatically.
Installing and Using debugpy
To set up debugpy for command line use, it can be installed via the command ‘Python -m pip install –upgrade debugpy’. This tool integrates seamlessly with Visual Studio Code and other IDEs, providing a comprehensive debugging environment for Python development.
Profiling and Performance Analysis
Profiling and performance analysis are critical components of debugging, helping developers identify performance bottlenecks and optimize their code. By using profiling tools, developers can pinpoint the slowest parts of their programs and make targeted improvements.
cProfile and profile Modules
The main built-in modules for profiling in Python are cProfile and profile. cProfile is designed to track the performance of function calls and generates a detailed report of function calls, including the execution time for each function. It has lower overhead compared to the profile module, making it preferable for large applications.
The profile module, on the other hand, is suitable for scenarios requiring more fine-grained profiling than cProfile can offer. Both tools are essential for identifying performance issues and optimizing Python programs.
Visualizing Profiling Data
Visualizing profiling data can provide deeper insights into performance issues. Tools like Snakeviz are used for visualizing profiling results interactively. Converting profiling data into flame graphs allows developers to easily identify performance bottlenecks and optimize their code accordingly.
Common Debugging Challenges and Solutions
Debugging often involves tackling common challenges such as SyntaxErrors, IndentationErrors, ImportErrors, and ModuleNotFoundErrors. Understanding these errors and their solutions is crucial for effective debugging.
Handling SyntaxErrors and IndentationErrors
SyntaxErrors typically occur due to invalid syntax, such as missing parentheses or misplaced colons, while IndentationErrors arise from inconsistent or incorrect indentation. To fix a SyntaxError, check for any missing syntax elements. Also, ensure that quotes, parentheses, and brackets are properly paired.
For IndentationErrors, ensure that your code uses consistent indentation using either spaces or tabs, but not both. Proper formatting is crucial in Python, as even minor inconsistencies can lead to these types of errors.
Fixing ImportErrors and ModuleNotFoundErrors
ImportErrors and ModuleNotFoundErrors occur when trying to load modules that are not installed or are incorrectly referenced. To fix these errors, verify that the necessary modules are installed using pip and ensure that the file paths are accurate.
Double-check the module names and paths to prevent these errors and ensure smooth module imports.
Summary
In summary, debugging is a vital skill for Python developers, encompassing a range of tools and techniques from basic command-line debugging to advanced methods like remote and post-mortem debugging. Popular Python debuggers such as PDB, PyCharm, and Visual Studio Code provide powerful features to help developers identify and fix issues efficiently. By leveraging these tools and adopting best practices, developers can enhance their debugging process, improve code quality, and make more informed decisions. Embrace these techniques and tools to become a more effective and confident Python developer.
Frequently Asked Questions
What is the significance of debugging in Python development?
Debugging is essential in Python development as it identifies and resolves errors, ensuring smooth and efficient program execution. This process enhances output verification, optimizes performance, and ultimately improves overall code quality.
How does PDB help in debugging Python code?
PDB aids in debugging Python code by enabling developers to pause execution, set breakpoints, and inspect variable values, thereby providing insight into the program's state at critical moments. This functionality enhances the debugging process significantly.
What are the benefits of using PyCharm for debugging?
Using PyCharm for debugging significantly enhances the development process through advanced features like step-by-step execution, variable inspection, breakpoints, and remote debugging. These tools cater to both beginners and experienced developers, facilitating more efficient and effective debugging.
How can I use Visual Studio Code for remote debugging?
You can use Visual Studio Code for remote debugging by configuring the launch.json file for your project and establishing an SSH tunnel for secure connections to the remote computer, allowing you to step through the program as it runs.
What tools are available for profiling and performance analysis in Python?
cProfile and the profile modules are essential for profiling Python programs, as they identify performance bottlenecks and generate detailed reports. Additionally, visualization tools such as Snakeviz can transform profiling data into flame graphs, facilitating easier analysis.