Mastering Python Metaclasses for Effective Object-Oriented Design
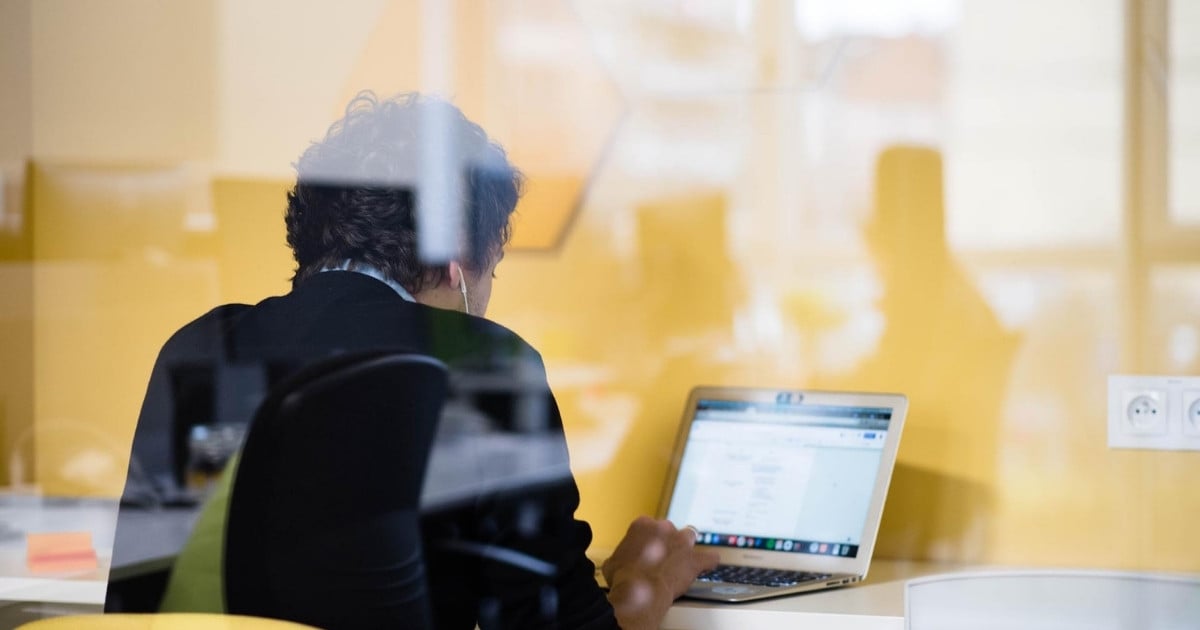
Key Takeaways
-
Metaclasses in Python serve as class factories, allowing developers to customize class creation and behavior beyond the capabilities of standard classes.
-
Custom metaclasses enable the enforcement of class constraints, the dynamic addition of methods and attributes, and facilitate the creation of domain-specific languages, enhancing code maintainability and flexibility.
-
While powerful, metaclasses should be used judiciously to avoid complexity; thorough documentation and awareness of common pitfalls are essential for effective implementation.
Understanding Python Metaclasses
Before delving into the power of metaclasses, it’s important to understand what they are and why they matter. In Python, metaclasses function as class factories; they create classes just as classes create instances. While classes define the behavior of objects, metaclasses shape the behavior of classes themselves.
Every class in Python is derived from a type, making type the default metaclass. This unification of classes and types in Python 3 means that all classes are new-style classes. When Python creates a new class, it uses a metaclass to do so, even if you don’t explicitly define one. This makes the type metaclass a fundamental part of Python’s class creation process.
Most Python programmers rarely deal directly with metaclasses. Tim Peters, a well-known Python developer, points out that simpler solutions are often preferable to using metaclasses. However, understanding metaclasses is key for grasping Python’s class internals and can be extremely useful for advanced developers.
Custom metaclasses enable developers to define specific behaviors and attributes for new class definitions.
By creating your own metaclass, you can control:
-
class creation
-
attributes
-
methods
-
behavior
The built-in type() function allows for dynamic class creation, offering developers a robust tool for customizing their class creation process.
Metaclasses in Python are a powerful yet often underutilized feature, providing a means to control and customize class creation and behavior. For advanced developers, understanding and leveraging metaclasses can lead to more flexible, robust, and maintainable Python applications.
Defining Custom Metaclasses
The true power of metaclasses emerges when defining custom ones. A metaclass in Python dictates how classes are created and their behavior. While the default metaclass, type, handles most class creation needs, sometimes customization is necessary to solve specific issues like altering class names or base classes.
To define a custom metaclass, create a new class that inherits from type. This inheritance allows you to control the class creation process and customize the behavior of the resulting class object. Let’s look at an example:
class MyMeta(type):
def __new__(cls, name, bases, dct):
dct['custom_attribute'] = 'This is custom!'
return super().__new__(cls, name, bases, dct)
class MyClass(metaclass=MyMeta):
pass
print(MyClass.custom_attribute) # Output: This is custom!
In this example, the new method is overridden to modify the class attributes during the class creation process. The new method is crucial as it allows for customized behavior when instantiating the class. If you do not define the init method in your custom metaclass, the default behavior of the type's init is applied.
Custom metaclasses can enforce constraints and ensure certain attributes or methods are present in the classes they create, which is useful for maintaining a consistent class structure across a codebase. By reassigning the new method, you can process and validate during class creation, ensuring the created class object meets specific criteria.
Defining custom metaclasses grants control over the class creation process, customization of class behavior, and enforcement of constraints, leading to more robust and maintainable code by ensuring classes adhere to specific requirements and behaviors.
Adding Class Attributes and Methods Dynamically
A powerful feature of metaclasses is their ability to dynamically add attributes and methods to classes, allowing for significant customization and flexibility during class creation. By altering the class’s namespace, metaclasses enable dynamic addition of attributes.
Attributes added via metaclasses are accessible to all instances of the class and its subclasses. This means that any changes made to the class structure using a metaclass will be inherited by all derived classes, ensuring consistency and reducing the need for redundant code.
For example, consider the following code:
class DynamicMeta(type):
def __new__(cls, name, bases, dct):
dct['dynamic_method'] = lambda self: 'Dynamic method called!'
return super().__new__(cls, name, bases, dct)
class DynamicClass(metaclass=DynamicMeta):
pass
instance = DynamicClass()
print(instance.dynamic_method()) # Output: Dynamic method called!
In this example, a new method, dynamic_method, is added to the class during its creation. This method is then accessible to all instances of the class, demonstrating how metaclasses can modify classes at creation time to add, delete, or rename methods.
Leveraging metaclasses to dynamically add class attributes and methods enables developers to create more flexible and maintainable code. This approach allows customization of class behavior without directly modifying class definitions, promoting a cleaner and more modular codebase.
Enforcing Class Constraints
Metaclasses can enforce specific constraints on classes, ensuring adherence to certain rules and structures, which is particularly useful in large codebases where consistency is crucial. They can enforce rules like requiring specific attributes in classes.
For instance, if you want to enforce that all classes in a project have a specific attribute, a metaclass can raise a TypeError if the required attribute is missing. This ensures that classes meet specified criteria before creation.
Here’s an example:
class ConstraintMeta(type):
def __new__(cls, name, bases, dct):
if 'required_attribute' not in dct:
raise TypeError(f"{name} is missing required attribute 'required_attribute'")
return super().__new__(cls, name, bases, dct)
class ValidClass(metaclass=ConstraintMeta):
required_attribute = 'I am here!'
# Uncommenting the following class would raise a TypeError
# class InvalidClass(metaclass=ConstraintMeta):
# pass
In this example, the ConstraintMeta metaclass checks for the presence of required_attribute during class creation. If the attribute is missing, a TypeError is raised, preventing the class from being created.
Enforcing constraints with metaclasses helps maintain a consistent class structure throughout your codebase, ensuring that classes behave as expected and adhere to required specifications. This approach is particularly beneficial in large projects or frameworks where consistency and reliability are essential.
Creating Domain-Specific Languages with Metaclasses
Domain-specific languages (DSLs) are specialized languages for specific application domains. Metaclasses play a key role in DSLs by translating custom syntax into executable Python code, allowing developers to create new, more intuitive syntaxes for specific tasks.
By defining a new syntax for classes, metaclasses can implement a DSL. For example, a DSL for defining state machines can use metaclasses to automatically set up necessary attributes for descriptors during class creation, ensuring ease of use and robustness.
Here’s a simple example:
class DSLMeta(type):
def __new__(cls, name, bases, dct):
dct['dsl_method'] = lambda self: 'DSL method called!'
return super().__new__(cls, name, bases, dct)
class DSLClass(metaclass=DSLMeta):
pass
dsl_instance = DSLClass()
print(dsl_instance.dsl_method()) # Output: DSL method called!
In this example, the DSLMeta metaclass adds a method to the class during its creation. This method is then accessible to all instances of the class, demonstrating how metaclasses can customize class creation to support the attributes and methods required for a DSL.
Enhancing Classes with Decorators and Annotations
Metaclasses can be combined with decorators to add functionality beyond standard class definitions, allowing for greater flexibility and modularity in class construction compared to traditional metaclass definitions.
When using decorators with metaclasses, copying existing class attributes is crucial to prevent them from appearing missing. Including the wrapped attribute can also assist with introspection in decorated classes.
Here’s an example:
def class_decorator(cls):
cls.decorated_method = lambda self: 'Decorated method called!'
return cls
@class_decorator
class DecoratedClass:
pass
decorated_instance = DecoratedClass()
print(decorated_instance.decorated_method()) # Output: Decorated method called!
In this example, the class_decorator function adds a method to the class during its creation. This method is then accessible to all instances of the class, demonstrating how decorators can enhance class functionality.
Dynamic Class Creation Using type()
The type() function in Python can dynamically create classes, providing a powerful alternative to traditional class definitions and allowing developers to create Python classes at runtime, enabling more flexible programming patterns.
The type() function can create a new class by calling it with three arguments: the class name, a tuple of base classes, and a dictionary containing class attributes and methods. This approach allows for the dynamic definition of class attributes and methods, enabling class creation customization.
Here’s an example:
DynamicClass = type('DynamicClass', (object,), {
'dynamic_method': lambda self: 'Dynamic method called!'
})
dynamic_instance = DynamicClass()
print(dynamic_instance.dynamic_method()) # Output: Dynamic method called!
In this example, a new class is created dynamically using the type() function. The class is defined with a class method, dynamic_method, which is then accessible to all instances of the class and demonstrates the use of class methods.
Practical Use Cases for Metaclasses
Metaclasses are often employed in libraries and frameworks requiring advanced customization and class manipulation. One primary use case is creating classes that meet an API’s requirements, ensuring consistent interfaces across implementations, which is crucial in large projects.
For example, metaclasses can enforce constraints during class creation by ensuring required attributes or methods are defined, raising errors for missing elements. This helps maintain a consistent and reliable codebase by ensuring all classes adhere to specified requirements.
Another practical use case for metaclasses is adding methods or attributes to classes, enhancing functionality without directly modifying definitions. This approach implements shared behavior across all instances of derived classes, streamlining maintenance and consistency.
Metaclasses are used in libraries and frameworks requiring advanced customization, such as the Django ORM. By employing metaclasses, these libraries can offer powerful and flexible APIs while maintaining a consistent and reliable codebase.
Metaclasses offer a powerful tool for creating consistent and reliable classes in large projects. By enforcing constraints, adding methods and attributes, and implementing shared behavior, metaclasses help maintain a consistent and maintainable codebase.
Common Pitfalls and How to Avoid Them
While metaclasses offer powerful customization capabilities, they can introduce additional complexity and potential pitfalls. One common mistake is creating custom metaclasses unnecessarily when simpler solutions suffice. Tim Peters, a prominent Python developer, advises that simpler solutions are usually preferable and that metaclasses should be used sparingly.
Metaclasses can make code harder to read and maintain, especially for developers unfamiliar with their intricacies. To avoid this, document the use of metaclasses thoroughly and make their purpose and functionality clear to other developers.
Inheriting from non-class types, like functions, can cause errors related to metaclasses. To avoid these, ensure all base classes are proper class types and that metaclasses are used appropriately.
Metaclass conflicts can occur when multiple base classes have different metaclasses. Resolving these conflicts may involve creating a custom metaclass that combines the metaclasses of the base classes. Python’s type() function can assist in managing metaclass resolution, facilitating conflict-free class creation.
Using a set to manage metaclasses may disrupt their order; employing a list can help maintain this order. By following these guidelines, developers can avoid common pitfalls and effectively leverage the power of metaclasses in their projects.
Summary
Metaclasses in Python provide a powerful tool for customizing class creation and behavior. By understanding and leveraging metaclasses, developers can create more flexible, robust, and maintainable code. From defining custom metaclasses to dynamically adding attributes and methods, enforcing class constraints, and creating domain-specific languages, metaclasses offer a wide range of possibilities for advanced Python developers.
While metaclasses can introduce additional complexity, they also provide a way to enforce consistent class structures, implement shared behavior, and create powerful and flexible APIs. By following best practices and being mindful of potential pitfalls, developers can effectively harness the power of metaclasses to create more elegant and maintainable code.