Top Tips to Boost React Native Performance in 2025
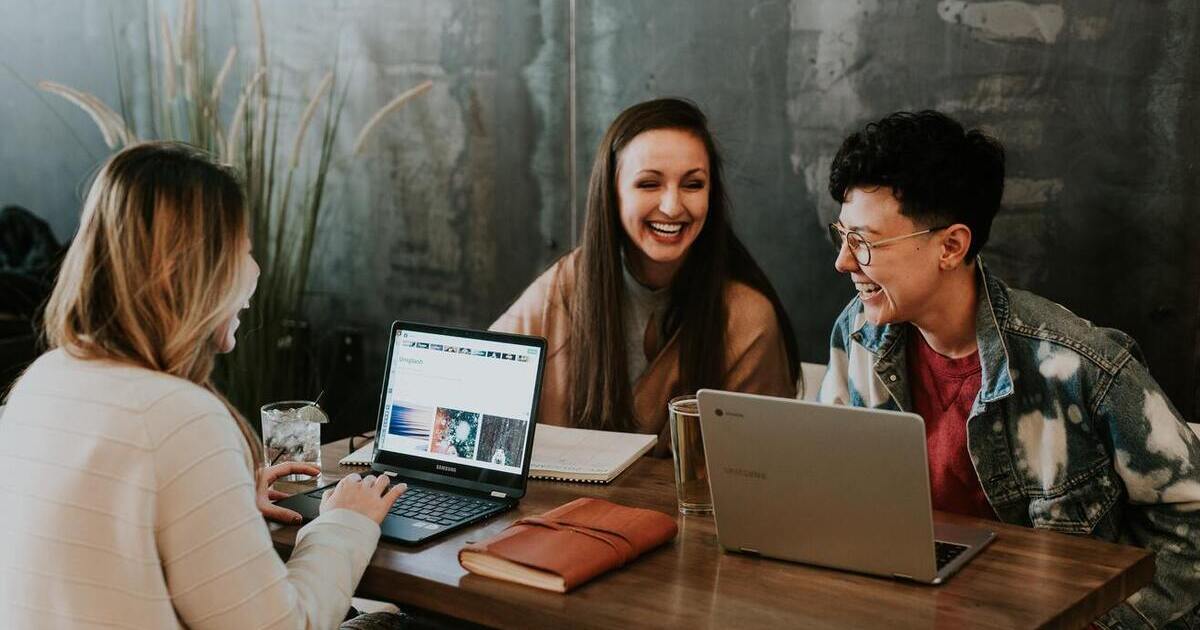
This article will discuss essential tips and techniques to boost your React Native app’s performance in 2025, making your application smoother and more responsive.
Key Takeaways
- Understanding the roles of the JavaScript and UI threads is essential for optimizing performance in React Native app development.
- Tools like PureComponent, React.memo(), and efficient image handling methods can significantly enhance rendering performance and reduce memory usage.
- Implementing efficient navigation strategies and leveraging Hermes can lead to faster load times and improved responsiveness in React Native applications.
Understanding React Native Performance
Optimal performance is the backbone of any successful app, providing users with a smooth and engaging experience. For React Native apps, achieving this involves a delicate balance between JavaScript execution and native performance. While React Native aims to deliver a minimum of 60 frames per second for fluid interactions, manual optimizations are often necessary to fine-tune the app’s performance.
Understanding the roles of the JavaScript thread and the main/UI threads is essential. Each plays a distinct part in rendering and user interactions, and optimizing their performance can significantly enhance the overall efficiency of your React Native application. Let’s delve deeper into these components.
JavaScript Thread vs. Native Code
The JavaScript thread in React Native manages business logic, API calls, and touch events. Its performance is critical; even brief unresponsiveness can cause dropped frames and disrupt the user experience.
Each frame at 60 frames per second must be generated in about 16.67 milliseconds, highlighting the need for a responsive JavaScript thread. Hermes, a JavaScript engine tailored for mobile apps, enhances performance by optimizing JavaScript execution through ahead-of-time compilation, leading to faster startup times and more efficient execution.
Balancing native code and JavaScript execution is essential. The React Native bridge enables seamless interaction between JavaScript business logic and native components, maintaining native performance standards.
Main Thread and UI Thread
Rendering and user interactions in React Native apps rely heavily on the main and UI threads. The main thread manages tasks like scrolling and animations, which must remain smooth for a responsive interface. Heavy operations on these threads can cause frame drops and janky animations, degrading the user experience.
Extensive rendering during UI transitions can cause noticeable lags. Minimizing heavy setState operations and using tools like StyleSheet for styling can help maintain smooth performance.
Optimizing these threads is crucial for a high-performing React Native app.
Common Performance Issues in React Native Apps
React Native applications, like any complex software, are susceptible to performance issues. Common problems include high memory usage and unnecessary re-renders, which can significantly degrade the app’s performance. Addressing these issues is essential for creating a high-performance application.
High Memory Usage
High memory usage is a frequent culprit behind sluggish React Native apps. Overusing rasterization properties can lead to increased memory consumption, memory leaks, and higher CPU usage on Android devices, impacting performance.
React Native generally consumes less memory than frameworks like Flutter on iOS devices, aiding smoother performance. Using the Hermes engine, with its efficient garbage collector, can further improve memory management and reduce consumption.
Unnecessary Re-renders
Unnecessary re-renders can cause performance bottlenecks in React Native applications. Arrow functions are used as callbacks, and anonymous functions in render methods create new instances, leading to frequent re-renders, especially in complex applications.
Implementing immutable data structures can improve change detection, minimizing unnecessary re-renders. Additionally, using tools like React.memo() and PureComponent can optimize component rendering by ensuring only necessary updates occur.
Optimizing Rendering and Re-rendering
Optimizing rendering and re-rendering is crucial for efficient React Native applications. Refining rendering strategies, such as minimizing app size and optimizing code, can significantly enhance performance.
Here, we explore two key techniques to achieve this.
Using PureComponent and React.memo()
PureComponent and React.memo() are powerful tools for optimizing component rendering. PureComponent automatically implements shouldComponentUpdate, allowing it to skip rendering when props and state haven’t changed. This makes it ideal for preventing unnecessary updates in class components.
React.memo() serves a similar purpose for functional components by memoizing them and comparing previous and current props to determine if a re-render is necessary. Using these tools together can lead to more efficient rendering and improved performance in React Native apps.
Avoiding Inline Functions as Props
Inline functions as props commonly cause performance issues in React Native. Each render creates a new function instance, leading to frequent and unnecessary re-renders. Arrow functions as callbacks and anonymous functions in render methods exacerbate this problem.
Developers should use bound methods or define functions outside of the render method to counteract this. This approach reduces the creation of new function instances, prevents unnecessary updates, and enhances performance.
Efficient Image Handling
Efficient image handling is essential for maintaining high performance in React Native apps. Unoptimized images can lead to high memory usage and slow loading times. Effective strategies to optimize images for scaling, resizing, and caching can significantly enhance app performance.
Scaling and Resizing Images
Optimizing images is crucial to prevent crashes and enhance performance in React Native apps. Unoptimized images can cause memory overload, leading to crashes. Adjusting the width or height of an Image component can be expensive for large images, as it involves re-cropping and scaling from the original image.
Using the style property transform with scale for animations can be more efficient. Additionally, the WebP format offers superior compression and quality compared to JPEG and PNG, making it a better choice for image optimization.
Caching Images with Third-Party Libraries
Caching images locally enhances performance by reducing network load. Libraries like react-native-fast-image simplify the caching process and improve loading efficiency. Proper image caching, including the use of a static image, prevents repeated downloads, ensuring faster load times and a smoother user experience.
Using third-party libraries for image caching not only optimizes performance but also simplifies the implementation, making it easier to manage and maintain the app’s efficiency.
Navigation Strategies for Better Performance
Effective navigation strategies are crucial for high-performing React Native apps. Leveraging tools like React Navigation and FlatList, developers can create seamless and efficient navigation experiences that enhance overall app performance.
Using React Navigation
React Navigation is the go-to library for managing navigation in React Native applications. It supports performance optimizations through its native stack navigator, which uses native stack views to improve performance. This ensures smooth and responsive navigation transitions, enhancing the user experience.
Adopting React Navigation allows developers to take advantage of its robust features and performance enhancements, making it an essential tool for creating high-performance React Native apps.
FlatList for Large Data Sets
Handling large data sets efficiently is essential for maintaining app performance. FlatList renders only visible items, significantly reducing memory usage and improving performance. It lazily loads items, ensuring an app remains responsive even with extensive data.
The getItemLayout function can enhance rendering speed by providing fixed heights for items, allowing faster and more efficient rendering. The lazy loading of items in FlatList helps minimize memory usage, making it an ideal choice for high-performance applications.
Enhancing Animation Performance
Smooth animations are crucial for a polished user experience. With tools like the nativeDriver and transformations, you ensure their animations are fluid and responsive, avoiding dropped frames and unresponsive UI.
Using nativeDriver with Animated API
Using the nativeDriver in animations bypasses the JavaScript thread, leading to smoother execution and a more fluid animation experience. Sending animations over the native bridge allows developers to achieve native performance standards.
To utilize the native driver, include ‘useNativeDriver: true’ in the animation configuration. This is particularly effective for non-layout properties like transform and opacity, ensuring efficient and responsive animations.
Transformations for Efficient Animations
Transformations allow for more efficient animations by changing the appearance without re-rendering the component. Transformations like scaling is particularly beneficial for larger images, as it is less resource-intensive than adjusting component dimensions.
Leveraging these techniques allows developers to create animations that are both visually appealing and performance-efficient, enhancing the overall user experience.
Improving Network Request Efficiency
Optimizing network request efficiency is essential for reducing latency and improving app responsiveness. Implementing strategies to minimize API calls and effectively cache network responses can significantly enhance performance.
Reducing API Calls
Minimizing API calls is crucial for improving performance in React Native applications. Efficient data retrieval methods and debouncing techniques can lessen the frequency of API calls in response to user actions, enhancing app responsiveness.
Managing API calls effectively reduces latency and improves the overall user experience, making the app more efficient and responsive.
Caching Network Responses
Caching network responses locally can significantly boost loading speeds and reduce server requests. Storing previously fetched data minimizes redundant network requests, leading to faster application response times and a better user experience.
Effective caching strategies should consider cache duration to maintain data freshness, ensuring the app remains responsive and efficient while providing up-to-date information.
Leveraging Hermes for Performance Gains
Utilizing Hermes can significantly enhance the performance of React Native applications. This open-source JavaScript engine is optimized for mobile apps, providing faster startup times and efficient memory management. Leveraging Hermes helps developers achieve smaller app sizes and improved execution speed, making their apps more responsive and efficient.
Enabling Hermes in React Native Projects
Enabling Hermes in React Native projects is straightforward. For Android, developers need to open the build.gradle file and add a specific configuration line. For iOS, the process involves modifying the Podfile with a similar configuration line. Hermes has been available for Android since version 0.60.4 and iOS since version 0.64-rc.0, making it accessible for most React Native projects.
Incorporating Hermes allows developers to take advantage of its performance benefits, leading to faster and more efficient apps. Ensuring Hermes is enabled in your project setup is a crucial step in optimizing your React Native application’s performance.
Benefits of Using Hermes
The benefits of using Hermes are substantial. They include:
- Significantly reducing the APK size of React Native apps, which is particularly beneficial for users with limited storage space.
- Optimizing launch speed.
- Minimizing memory usage, resulting in a smoother and more responsive user experience.
These advantages make Hermes an essential tool for developers aiming to build high-performance React Native applications.
Profiling and Debugging Performance
Profiling and debugging are crucial for identifying and resolving performance issues in React Native apps. Using tools and techniques to analyze resource usage and optimize the JavaScript thread ensures applications run smoothly across various devices.
Using Flipper for Debugging
Flipper is a powerful debugging platform that supports React Native. It provides developers with insights into app performance and helps speed up the debugging process. Utilizing Flipper allows developers to reduce bugs and improve the overall performance of their React Native applications.
Firebase Performance Monitoring
Firebase Performance Monitoring is a valuable tool for gaining insights into app performance. Developers can review and analyze performance data collected from their app in the Firebase console, identifying and addressing performance bottlenecks effectively.
This comprehensive monitoring helps ensure the app’s performance remains efficient and responsive.
Summary
In conclusion, optimizing the performance of React Native apps is crucial for delivering a smooth and responsive user experience. By understanding the roles of the JavaScript and main/UI threads, addressing common performance issues, and implementing strategies for efficient rendering, image handling, navigation, animations, and network requests, developers can significantly enhance their applications.
Leveraging tools like Hermes, Flipper, and Firebase Performance Monitoring can further boost performance, ensuring that React Native apps meet the high standards of today’s mobile users. By following these best practices and continuously monitoring and optimizing performance, developers can create high-performance applications that delight users and stand out in the competitive app market.
Frequently Asked Questions
What is React Native?
React Native is a framework developed by Facebook that allows developers to create natively rendered applications using JavaScript. This enables cross-platform development while maintaining a native look and feel.
How does React Native compare to other frameworks in terms of memory usage?
React Native typically demonstrates lower memory consumption compared to frameworks such as Flutter on iOS devices, leading to enhanced performance. This efficiency makes it an attractive choice for developers concerned about resource management.
What is Hermes, and how does it benefit React Native apps?
Hermes is an open-source JavaScript engine designed specifically for mobile applications, benefiting React Native apps by enhancing performance through reduced APK size, faster launch speeds, and lower memory consumption.
How can I enable Hermes in my React Native project?
To enable Hermes in your React Native project, modify the `build.gradle` file for Android and the Podfile for iOS by adding the necessary configuration lines. This will allow you to take advantage of Hermes for improved performance.
What tools can I use to monitor and debug performance in React Native apps?
To monitor and debug performance in React Native apps, Flipper and Firebase Performance Monitoring are highly recommended for their ability to provide valuable insights and identify bottlenecks effectively.